Customizing JavaFX labels with CSS styling
Write a JavaFX program that constructs a UI with labels. Use CSS to change the font family, size, color, and alignment of the text in the labels.
Sample Solution:
JavaFx Code:
// Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Styled Labels");
// Creating labels
Label label1 = new Label("Label 1");
Label label2 = new Label("Label 2");
Label label3 = new Label("Label 3");
// Setting IDs for labels to apply CSS styling
label1.setId("custom-label");
label2.setId("custom-label");
label3.setId("custom-label");
// Creating a VBox layout to hold the labels
VBox vbox = new VBox(12, label1, label2, label3);
vbox.getStyleClass().add("label-container");
// Create a scene with the VBox layout
Scene scene = new Scene(vbox, 300, 200);
// Load CSS file to style the labels
scene.getStylesheets().add(getClass().getResource("styles.css").toExternalForm());
// Set the scene on the stage and display it
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
/*styles.css*/
#custom-label {
-fx-font-family: "Arial";
-fx-font-size: 18px;
-fx-text-fill: #1E90FF; /* Blue text color */
-fx-alignment: center;
}
.label-container {
-fx-background-color: #f2f2f2; /* Light gray background */
-fx-padding: 10px;
}
Explanation:
In the exercise above the JavaFX code creates a simple application that displays three labeled elements vertically using a VBox layout. The labels are styled using CSS.
The code is explained briefly below:
- Setting up the UI: The start method initializes a JavaFX window (Stage) with the title "Styled Labels". Three labels (Label) labeled as "Label 1", "Label 2", and "Label 3" are created.
- Assigning IDs for CSS Styling: IDs are set for the labels using setId() method to allow applying CSS styling to these specific labels.
- Layout Structure: The labels are placed in a vertical box (VBox) layout with a spacing of 12 pixels between each label using the VBox constructor.
- CSS Styling: The scene (Scene) loads an external CSS file (styles.css) using getStylesheets().add() to apply styling to the labels based on their IDs.
- Launching the Application: The main method launches the JavaFX application by calling launch(args).
Sample Output:
Flowchart:
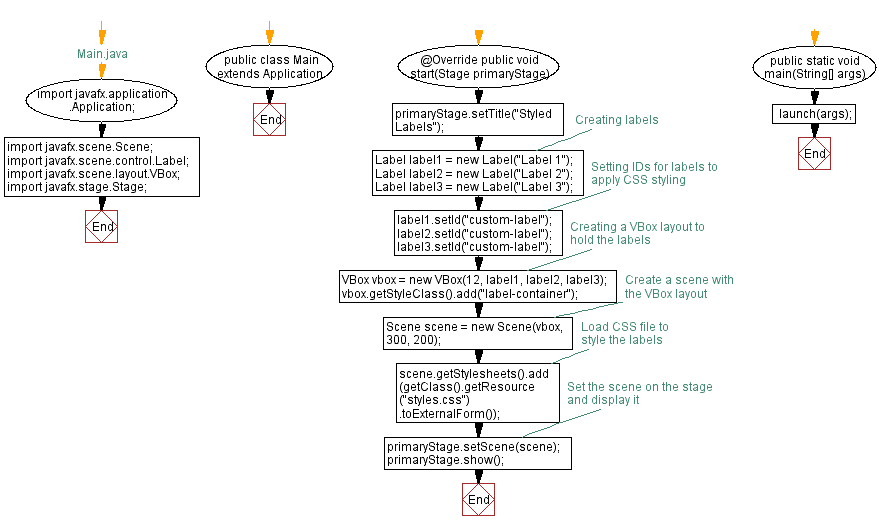
Java Code Editor:
Previous: Creating a JavaFX form with styled text fields.
Next: Customizing JavaFX toggle buttons with CSS styling.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.