Creating a JavaFX form with styled text fields
Write a JavaFX program that creates a form with text fields. Apply CSS styling to modify the appearance of the text fields, such as changing the border color, background, and text color.
Sample Solution:
JavaFx Code:
// Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Styled Form");
// Creating text fields
TextField textField1 = new TextField();
TextField textField2 = new TextField();
TextField textField3 = new TextField();
// Labels for text fields
Label label1 = new Label("Name:");
Label label2 = new Label("Email:");
Label label3 = new Label("Phone:");
// Creating a VBox layout to hold the form elements
VBox vbox = new VBox();
vbox.getChildren().addAll(
createFormField(label1, textField1),
createFormField(label2, textField2),
createFormField(label3, textField3)
);
// Create a scene with the VBox layout
Scene scene = new Scene(vbox, 300, 200);
// Load CSS file to style the text fields
scene.getStylesheets().add(getClass().getResource("styles.css").toExternalForm());
// Set the scene on the stage and display it
primaryStage.setScene(scene);
primaryStage.show();
}
// Helper method to create form fields with labels
private VBox createFormField(Label label, TextField textField) {
VBox vBox = new VBox();
vBox.getChildren().addAll(label, textField);
vBox.getStyleClass().add("form-field");
return vBox;
}
public static void main(String[] args) {
launch(args);
}
}
/*styles.css*/
.form-field .text-field {
-fx-border-color: #4CAF50; /* Green border */
-fx-background-color: #f2f2f2; /* Light gray background */
-fx-text-fill: #333333; /* Dark text color */
}
Explanation:
In the exercise above,
- Setting up the UI: The start method initializes a JavaFX window (Stage) with the title "Styled Form". It creates three text fields (TextField) and three labels (Label) to represent form elements for "Name," "Email," and "Phone."
- Layout Structure: The form elements are arranged in a vertical box (VBox) layout using the VBox class. The createFormField method pairs each label with its respective text field, creating a structure of labeled input fields.
- CSS Styling: The styles.css file contains CSS styling for form fields. It specifies a class selector .form-field .text-field to target text fields nested within elements with the class .form-field. This styling includes properties like border color, background color, and text color for the text fields within the form.
- Scene and Stylesheet: The scene (Scene) is created with the VBox layout, set to a size of 300x200 pixels. The CSS file is loaded into the scene using scene.getStylesheets().add() to apply the specified styles to the text fields within the form.
- Launching the Application: The main method launches the JavaFX application by calling launch(args).
Sample Output:
Flowchart:
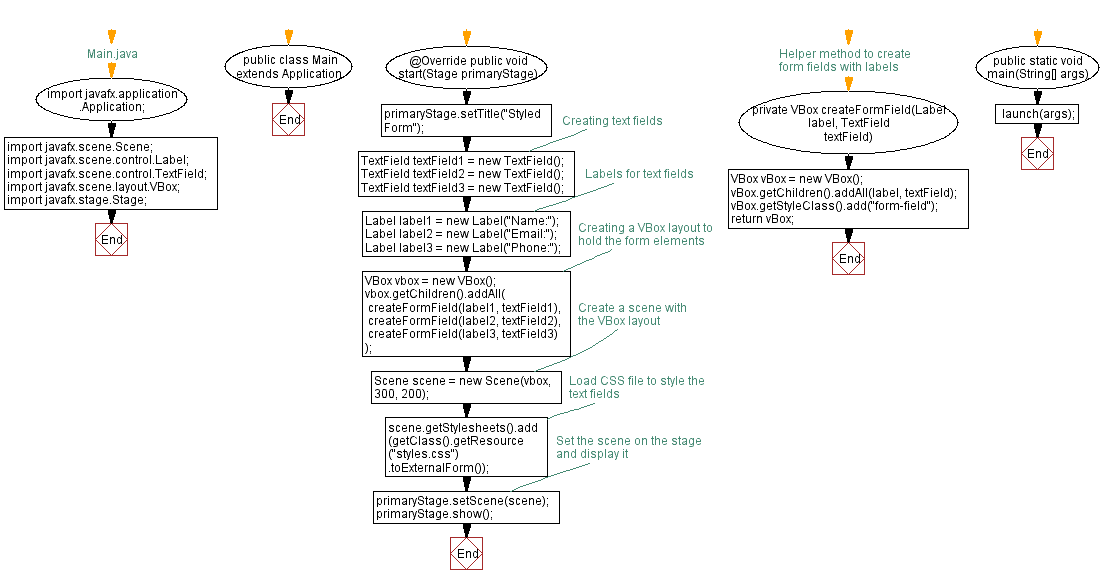
Java Code Editor:
Previous: JavaFX Application with multiple styled buttons.
Next: Customizing JavaFX labels with CSS styling.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.