Calculate factorial using Lambda expression in Java
Java Lambda Program: Exercise-8 with Solution
Write a lambda expression to implement a lambda expression to calculate the factorial of a given number.
Sample Solution:
Java Code:
import java.util.function.LongUnaryOperator;
public class Main {
public static void main(String[] args) {
// Define the factorial lambda expression
LongUnaryOperator factorial = n -> {
long result = 1;
for (long i = 1; i <= n; i++) {
result *= i;
}
return result;
};
// Calculate the factorial of a number using the lambda expression
long n = 7;
long factorial_result = factorial.applyAsLong(n);
// Print the factorial result
System.out.println("Factorial of " + n + " is: " + factorial_result);
}
}
Sample Output:
Factorial of 7 is: 5040
Explanation:
In the above exercise, we define a lambda expression using the LongUnaryOperator functional interface. This interface represents an operation on a single long operand and produces a long result.
In the main method, we create an instance of the LongUnaryOperator using the lambda expression. Then, we specify the number for which the factorial should be calculated.
In order to calculate the factorial, we use the applyAsLong method of the lambda expression and pass the number as an argument. In the factorialResult variable, we store the result.
Lastly, we print the factorial result using System.out.println ().
Flowchart:
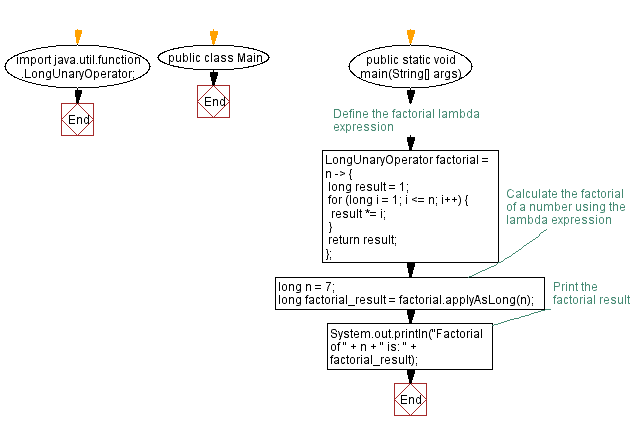
Live Demo:
Java Code Editor:
Improve this sample solution and post your code through Disqus
Java Lambda Exercises Previous: Remove duplicates from list of integers using Lambda expression in Java.
Java Lambda Exercises Next: Check prime number using Lambda expression in Java.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics