Java JUnit Test Case: Test Setup and Teardown
Java Unit Test: Exercise-3 with Solution
Write a Java test case that implements a JUnit test with setup and teardown methods to prepare and clean up resources for multiple test cases.
Sample Solution:
Java Code:
// ExceptionTest.java
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
import static org.junit.Assert.*;
public class SetupTeardownTest {
// Example class with setup and teardown operations
public class ExampleClass {
private String resource;
// Setup method - runs before each test
@Before
public void setUp() {
System.out.println("Setting up resources...");
resource = "Initialized Resource";
}
// Teardown method - runs after each test
@After
public void tearDown() {
System.out.println("Cleaning up resources...");
resource = null;
}
// Method to be tested
public String getResource() {
return resource;
}
}
// JUnit test case with setup and teardown
@Test
public void testResourceInitialization() {
// Arrange
ExampleClass example = new ExampleClass();
// Act
String result = example.getResource();
// Assert
assertEquals("Initialized Resource", result);
}
// JUnit test case with setup and teardown
@Test
public void testResourceCleanup() {
// Arrange
ExampleClass example = new ExampleClass();
// Act
example.getResource(); // Invoke the method to trigger setup and teardown
// Assert
assertNull(example.getResource());
}
// Main function to run JUnit tests
public static void main(String[] args) {
Result result = JUnitCore.runClasses(SetupTeardownTest.class);
// Check if there are any failures
if (result.getFailureCount() > 0) {
System.out.println("Test failed:");
// Print details of failures
for (Failure failure : result.getFailures()) {
System.out.println(failure.toString());
}
} else {
System.out.println("All tests passed successfully.");
}
}
}
Sample Output:
testResourceInitialization(SetupTeardownTest): expected:<Initialized Resource> but was:<null>
Explanation:
In the exercise above -
- The "ExampleClass" has @Before and @After annotated methods (setUp and tearDown) for setup and teardown operations.
- The "testResourceInitialization" and "testResourceCleanup" test cases use these setup and teardown methods.
- The main() function runs the JUnit tests using JUnitCore.runClasses(SetupTeardownTest.class).
Flowchart:
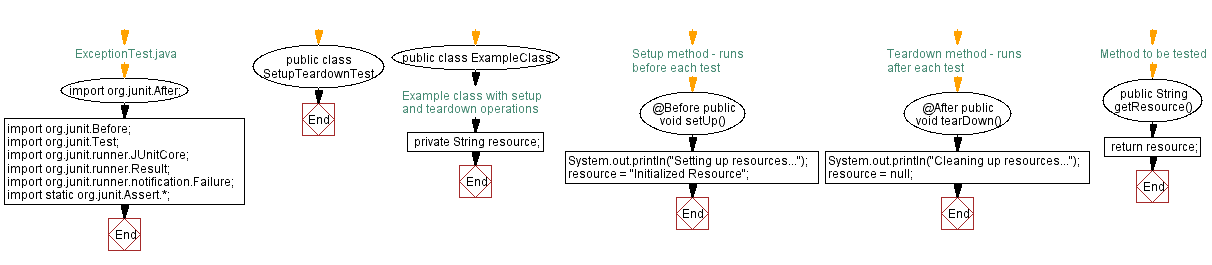
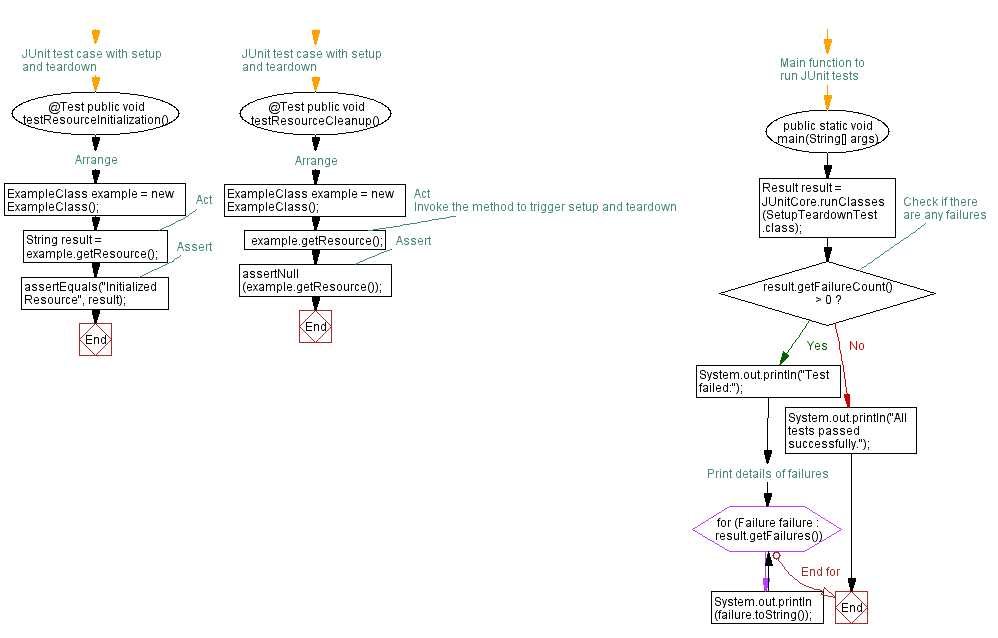
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Java Test Case: Exception Testing.
Next: Java Parameterized test with JUnit: MultiplyTest example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/unittest/java-unittest-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics