Java Parameterized test with JUnit: MultiplyTest example
Java Unit Test: Exercise-4 with Solution
Write a Java program that implements parameterized test to verify that a method behaves correctly for different input values.
Sample Solution:
Java Code:
// ParameterizedTest.java
import org.junit.Test;
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
import org.junit.runners.Parameterized;
import org.junit.runners.Parameterized.Parameters;
import org.junit.runner.RunWith;
import static org.junit.Assert.assertEquals;
import java.util.Arrays;
import java.util.Collection;
public class ParameterizedTest {
// Example class with the method to be tested
public static class ExampleClass {
public int multiply(int a, int b) {
return a * b;
}
}
// Parameterized test case
@RunWith(Parameterized.class)
public static class MultiplyTest {
private int input1;
private int input2;
private int expectedResult;
// Constructor with parameters
public MultiplyTest(int input1, int input2, int expectedResult) {
this.input1 = input1;
this.input2 = input2;
this.expectedResult = expectedResult;
}
// Parameters for the test cases
@Parameters
public static Collection
Sample Output:
All tests passed successfully.
Explanation:
The above Java code shows a parameterized JUnit test using the "Parameterized" runner. Here's a brief explanation:
- ExampleClass:
- Defines a simple class named "ExampleClass" with a method "multiply(int a, int b)" that multiplies two integers.
- MultiplyTest:
- A nested static class, "MultiplyTest", is marked with @RunWith(Parameterized.class). It signifies that this class will be run using the "Parameterized" runner.
- Constructor: It has a constructor with parameters (int input1, int input2, int expectedResult) that will be used to pass values for parameterized testing.
- @Parameters method: The "data()" method annotated with @Parameters provides a collection of parameter sets (arrays of inputs and expected results).
- Test Method: The "testMultiply()" method annotated with @Test is the actual JUnit test. It uses the provided parameters to perform the multiplication using the "ExampleClass" and asserts that the result matches the expected value.
- Main Function:
- The "main()" function is used to run the JUnit tests.
- JUnitCore.runClasses(MultiplyTest.class) runs the "MultiplyTest" class using the JUnitCore runner.
- The results are checked, and if there are any test failures, details are printed. Otherwise, a success message is displayed.
Flowchart:
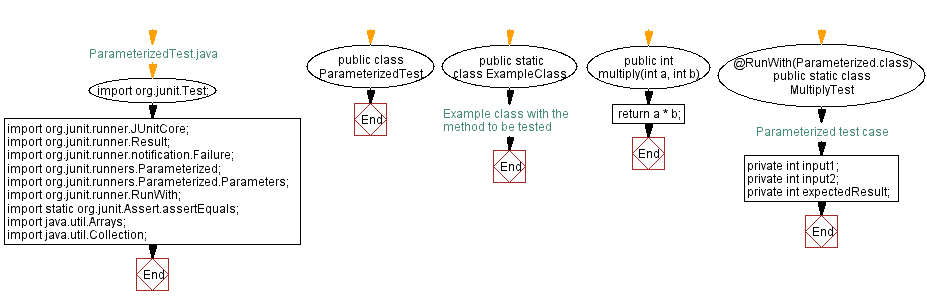
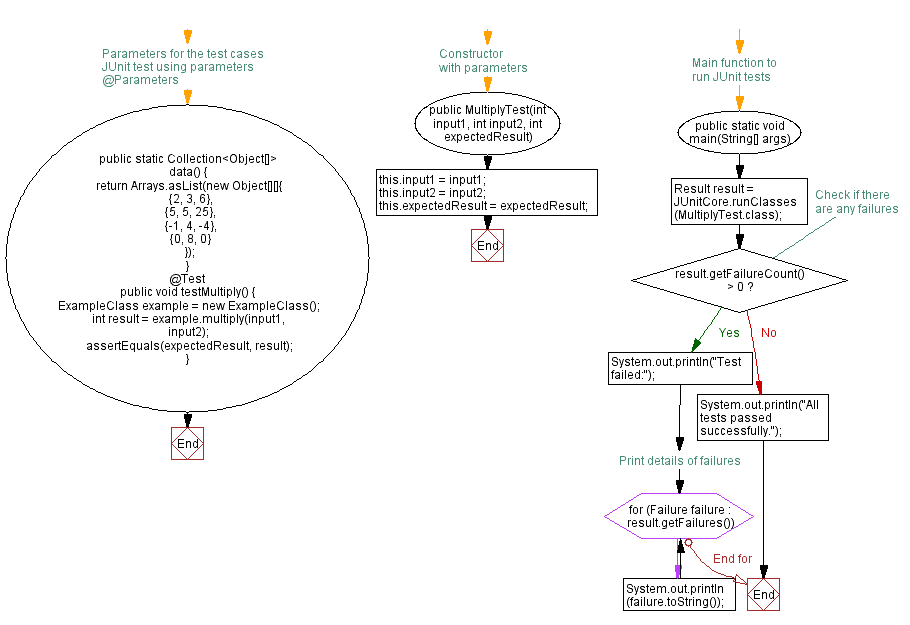
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Java JUnit Test Case: Test Setup and Teardow.
Next: Java Timeout test with JUnit: Timeout Test example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics