Java Timeout test with JUnit: Timeout Test example
5. Fail Test on Timeout
Write a Java program that implements parameterized test to verify that a method behaves correctly for different input values.
Sample Solution:
Java Code:
// TimeoutTest.java
import org.junit.Test;
import org.junit.Rule;
import org.junit.rules.Timeout;
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
public class TimeoutTest {
// Rule to set a timeout for all test methods in this class
@Rule
public Timeout globalTimeout = new Timeout(2); // Set the timeout in seconds
// Example class with the method to be tested
public static class ExampleClass {
public void longRunningMethod() {
// Simulate a long-running operation
try {
Thread.sleep(3000); // Sleep for 3 seconds
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
// JUnit test case with a timeout for the specific method
@Test(timeout = 2000) // Set the timeout in milliseconds
public void testLongRunningMethod() {
ExampleClass example = new ExampleClass();
example.longRunningMethod();
}
// Main function to run JUnit tests
public static void main(String[] args) {
Result result = JUnitCore.runClasses(TimeoutTest.class);
// Check if there are any failures
if (result.getFailureCount() > 0) {
System.out.println("Test failed:");
// Print details of failures
for (Failure failure : result.getFailures()) {
System.out.println(failure.toString());
}
} else {
System.out.println("All tests passed successfully.");
}
}
}
Sample Output:
Test failed: testLongRunningMethod(TimeoutTest): test timed out after 2 milliseconds
Explanation:
In the exercise above-
- @Rule and Timeout: The "globalTimeout" is a JUnit rule annotated with @Rule. It sets a global timeout for all test methods in the class. The timeout is specified in seconds using the "Timeout" rule.
- ExampleClass: This inner class represents a hypothetical class (ExampleClass) with a method (longRunningMethod) that simulates a long-running operation by sleeping for 3 seconds.
- @Test(timeout = 2000): The "testLongRunningMethod" method is a JUnit test case annotated with @Test. It tests the "longRunningMethod" and specifies a timeout of 2000 milliseconds (2 seconds) for this specific test.
- main Method: The main method is used to run the JUnit tests. It utilizes "JUnitCore.runClasses" to execute the tests and prints whether the tests passed or failed, along with details of any failures.
Flowchart:
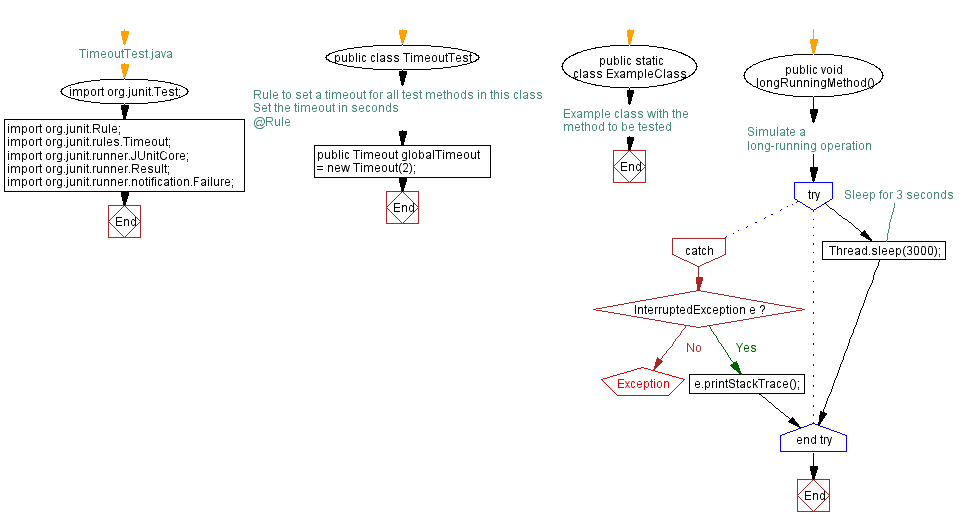
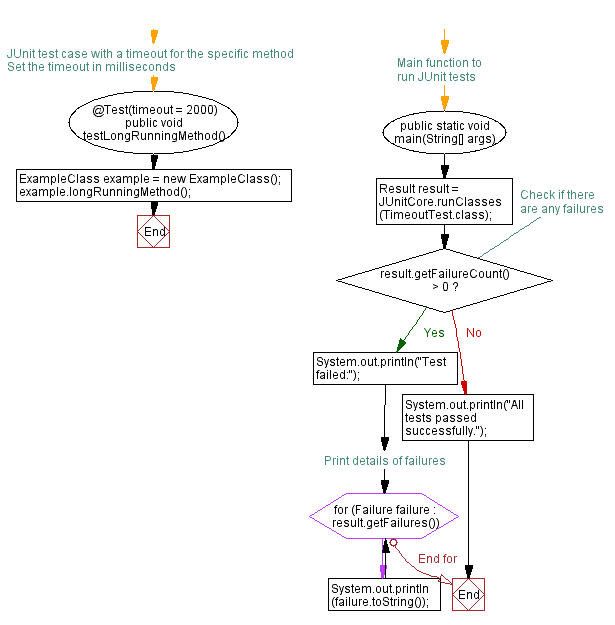
For more Practice: Solve these Related Problems:
- Write a Java program to create a test case using a timeout annotation that fails if a method does not complete within a specified time limit.
- Write a Java program to design a test case that asserts a long-running method finishes execution within an acceptable threshold.
- Write a Java program to implement a test case that measures method execution time and fails if the duration exceeds a predefined limit.
- Write a Java program to construct a test case that cancels a method call if it takes longer than the allocated time using JUnit's timeout feature.
Go to:
PREV : Parameterized Test for Multiple Inputs.
NEXT : Ignore Test Case Scenario.
Java Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.