Java ignored test Case with JUnit: IgnoredTest example
6. Ignore Test Case Scenario
Write a Java test case that is intentionally ignored, and explain the scenarios where test skipping might be useful.
Sample Solution:
Java Code:
// IgnoredTest.java
import org.junit.Test;
import org.junit.Ignore;
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
public class IgnoredTest {
@Test
public void testValidInput() {
// Test implementation for valid input
}
@Ignore("Test is ignored intentionally")
@Test
public void testInvalidInput() {
// Test implementation for invalid input
}
public static void main(String[] args) {
Result result = JUnitCore.runClasses(IgnoredTest.class);
// Check if there are any failures
if (result.getFailureCount() > 0) {
System.out.println("Test failed:");
// Print details of failures
for (Failure failure : result.getFailures()) {
System.out.println(failure.toString());
}
} else {
System.out.println("All tests passed successfully.");
}
}
}
Sample Output:
All tests passed successfully.
Explanation:
In the exercise above-
- @Ignore Annotation: The "testInvalidInput" method is annotated with @Ignore. This annotation indicates that the test is intentionally ignored, and it provides an optional message explaining why the test is ignored.
- Scenario where Test Skipping is Useful:
- Incomplete Implementation: When a specific test is not fully implemented or is known to be incomplete, it can be marked as ignored until the implementation is complete.
- Known Issues: If there are known issues with a particular test or if certain features are not yet available, the corresponding tests can be ignored until those issues are addressed.
- Work in Progress: During development, when various parts of the codebase are in progress, developers might choose to ignore tests related to unfinished or experimental features.
- Temporary Exclusion: In some scenarios, you might want to temporarily exclude a test from the test suite, perhaps to focus on other parts of the codebase without being distracted by known failing tests.
Flowchart:
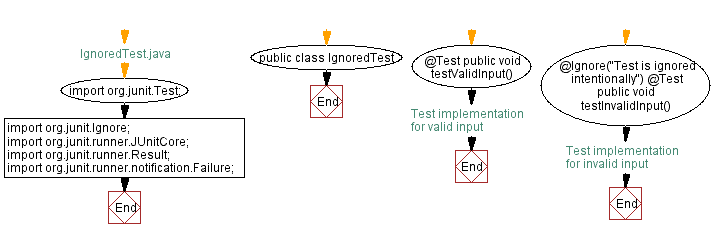
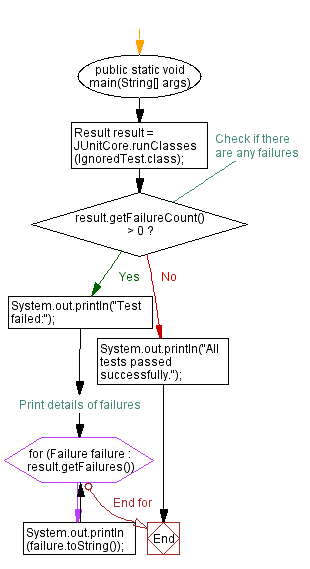
For more Practice: Solve these Related Problems:
- Write a Java program to create a test case marked with @Ignore that skips execution and provides a comment explaining the reason.
- Write a Java program to implement a test case that conditionally ignores tests based on a system property or configuration.
- Write a Java program to design a test case that is temporarily disabled due to incomplete functionality or external dependency issues.
- Write a Java program to construct a test case with @Ignore and a custom message to indicate why the test is currently not executed.
Go to:
PREV : Fail Test on Timeout.
NEXT : Assert with Custom Error Message.
Java Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.