Java Custom Assertion Example: Providing Meaningful feedback in tests
Java Unit Test: Exercise-7 with Solution
Write a Java program that uses assertions with custom error messages to provide meaningful feedback when a test fails.
Sample Solution:
Java Code:
// CustomAssertionTest.java
import org.junit.Test;
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
public class CustomAssertionTest {
@Test
public void testAddition() {
int result = add(2, 3);
assertWithMessage("Addition failed: ", result == 5);
}
@Test
public void testMultiplication() {
int result = multiply(2, 3);
assertWithMessage("Multiplication failed: ", result == 6);
}
private int add(int a, int b) {
return a + b;
}
private int multiply(int a, int b) {
return a * b;
}
private static void assertWithMessage(String message, boolean condition) {
assert condition : message;
}
public static void main(String[] args) {
Result result = JUnitCore.runClasses(CustomAssertionTest.class);
// Check if there are any failures
if (result.getFailureCount() > 0) {
System.out.println("Test failed:");
// Print details of failures
for (Failure failure : result.getFailures()) {
System.out.println(failure.toString());
}
} else {
System.out.println("All tests passed successfully.");
}
}
}
Sample Output:
All tests passed successfully.
Explanation:
In the exercise above-
- assertWithMessage Method: This method takes a custom error message and a boolean condition. It uses the "assert" statement to check the condition, and if the condition is false, it throws an "AssertionError" with the provided custom message.
- testAddition and testMultiplication Methods: These are JUnit test methods that use custom assertions with meaningful error messages.
- main Method: Runs the JUnit tests and prints whether the tests passed or failed. If a test fails, it prints details about the failures.
- add and multiply Methods: These are example methods that perform addition and multiplication, respectively.
When running this program, if a test fails, the custom error message specified in the "assertWithMessage" method will provide additional context about the failure.
Flowchart:
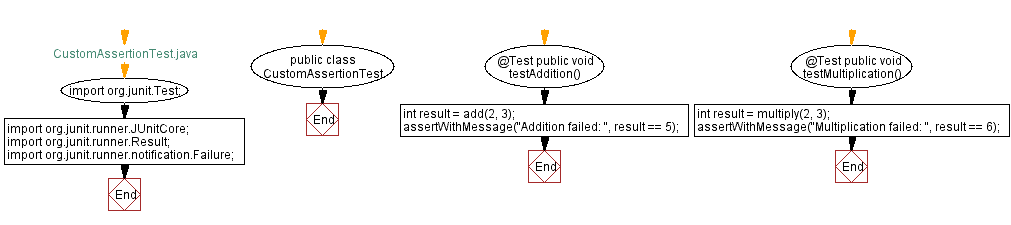
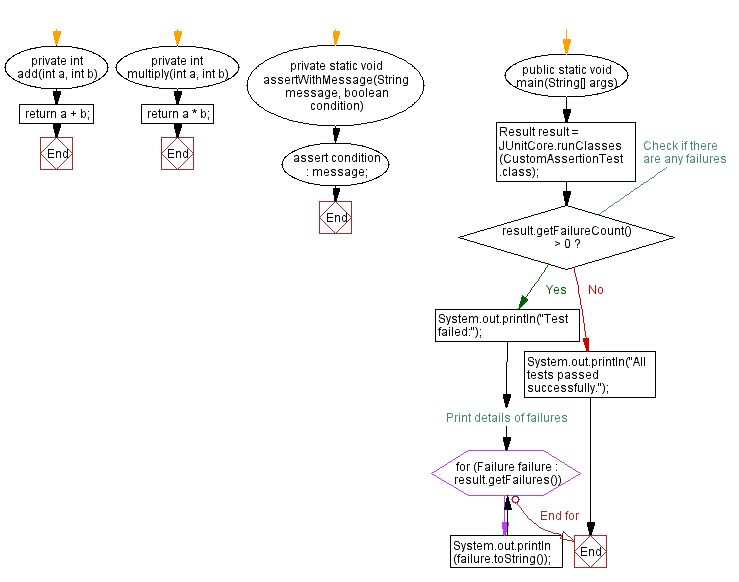
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Java ignored test Case with JUnit: IgnoredTest example.
Next: Java Testing Private methods with reflection: ExampleClass demonstration.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics