JavaScript HTTP GET Request with Promise
JavaScript Asynchronous: Exercise-3 with Solution
HTTP GET with Promises
Write a JavaScript a function that makes an HTTP GET request and returns a Promise that resolves with the response data.
Sample Solution:
JavaScript Code:
function make_Get_Request(url) {
return new Promise((resolve, reject) => {
fetch(url)
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.then(data => resolve(data))
.catch(error => reject(error));
});
}
make_Get_Request('https://example.com/data')
.then(data => {
console.log('Response data: ' + data);
})
.catch(error => {
console.log('Error: ' + error.message);
});
Output:
"Error:" "Failed to fetch"
Note: Executed on JS Bin
Explanation:
In the above exercise,
- The "make_Get_Request()" function accepts a URL as a parameter.
- Within the function, a new Promise is created using the Promise constructor. The executor function takes two parameters, resolve and reject, which control the Promise's state.
- Inside the Promise's executor function, the fetch function is called with the provided URL. The fetch function returns a Promise that resolves to the Response object representing the response to the request.
- The then method is chained to the fetch Promise to handle the response. In this step, we check if the response is not OK (status code other than 200). If it's not OK, we throw an error using throw new Error() with a custom message indicating the HTTP error.
- If the response is OK, we call response.json() to extract the JSON data from the response. This also returns a Promise.
- Another "then()" method is chained to handle the resolved data. In this step, we resolve the outer Promise created in make_Get_Request with the obtained data using resolve(data).
- If any error occurs during the process, the catch method is used to handle rejection. The error is passed to the reject function, causing the outer Promise to be rejected with the error.
- The make_Get_Request function returns the Promise created in step 2.
- Finally, the make_Get_Request function is called with a sample URL 'https://example.com/data'. The returned Promise is then used with .then() and .catch() to handle the resolved data or any error during the HTTP request.
Flowchart:
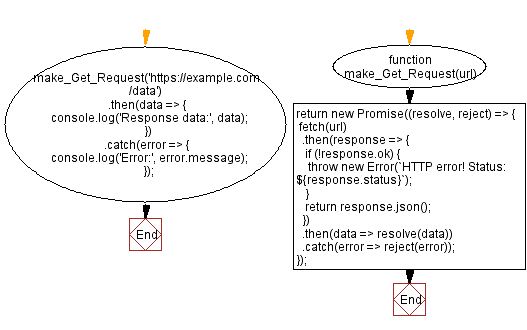
Live Demo:
See the Pen javascript-asynchronous-exercise-3 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that performs an HTTP GET request using XMLHttpRequest wrapped inside a Promise, resolving with the response text.
- Write a JavaScript function that uses the Fetch API to make an HTTP GET request and returns a Promise that resolves to parsed JSON data.
- Write a JavaScript function that implements an HTTP GET request with a Promise, rejecting if the HTTP status is not in the 200–299 range.
- Write a JavaScript function that makes an HTTP GET request using a Promise and includes a mechanism to time out if the request takes too long.
Improve this sample solution and post your code through Disqus.
Asynchronous Exercises Previous: Callback to Promise | Transforming asynchronous functions.
Asynchronous Exercises Next: Parallel URL Content Download with JavaScript Promises.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.