Parallel URL Content Download with JavaScript Promises
JavaScript Asynchronous: Exercise-4 with Solution
Parallel URL Downloads with Promises
Write a JavaScript function that takes an array of URLs and downloads the contents of each URL in parallel using Promises.
Sample Solution:
JavaScript Code:
function downloadContents(urls) {
const promises = urls.map(url => {
return new Promise((resolve, reject) => {
fetch(url)
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.text();
})
.then(data => resolve(data))
.catch(error => reject(error));
});
});
return Promise.all(promises);
}
// Usage example:
const urls = [
'https://jsonplaceholder.typicode.com/posts/1',
'https://jsonplaceholder.typicode.com/posts/2',
'https://jsonplaceholder.typicode.com/posts/3'
];
downloadContents(urls)
.then(contents => {
console.log('Downloaded contents:', contents);
})
.catch(error => {
console.log('Error:', error.message);
});
Output:
"Downloaded contents:" ["{ \"userId\": 1, \"id\": 1, \"title\": \"sunt aut facere repellat provident occaecati excepturi optio reprehenderit\", \"body\": \"quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto\" }", "{ \"userId\": 1, \"id\": 2, \"title\": \"qui est esse\", \"body\": \"est rerum tempore vitae\nsequi sint nihil reprehenderit dolor beatae ea dolores neque\nfugiat blanditiis voluptate porro vel nihil molestiae ut reiciendis\nqui aperiam non debitis possimus qui neque nisi nulla\" }", "{ \"userId\": 1, \"id\": 3, \"title\": \"ea molestias quasi exercitationem repellat qui ipsa sit aut\", \"body\": \"et iusto sed quo iure\nvoluptatem occaecati omnis eligendi aut ad\nvoluptatem doloribus vel accusantium quis pariatur\nmolestiae porro eius odio et labore et velit aut\" }"]
Note: Executed on JS Bin
Explanation:
In the above exercise -
- The "downloadContents()" function accepts an array of URLs as the urls parameter.
- It creates an array of Promises using the "map()" method on the urls array. Each Promise represents a fetch request to a specific URL.
- Inside each Promise, a fetch request is made to the corresponding URL using the "fetch()" function. It returns a Promise that resolves with the response.
- The first .then block checks if the response is successful (response.ok). If not, it throws an error with a custom message indicating HTTP status.
- If the response is successful, the second .then block extracts the response data using response.text(), which returns a Promise that resolves with the text content.
- Resolved data is then passed to the resolve function to fulfill the Promise.
- If any error occurs during the fetch request or response handling, the .catch block is executed. Errors are passed to the reject function, which rejects the Promise.
- The resulting array of Promises is returned from the downloadContents function using Promise.all, which returns a Promise that resolves to an array of fulfilled Promise values.
In the usage example, an array of URLs is defined, representing three different posts from the jsonplaceholder.typicode.com API.
- The downloadContents function is called with the urls array, initiating the parallel download of contents from the specified URLs.
- When all the Promises are fulfilled (i.e., when all the contents are downloaded), the .then block is executed, and the downloaded contents are logged to the console.
- If any error occurs during the process, the .catch block is executed, and the error message is logged to the console.
Flowchart:
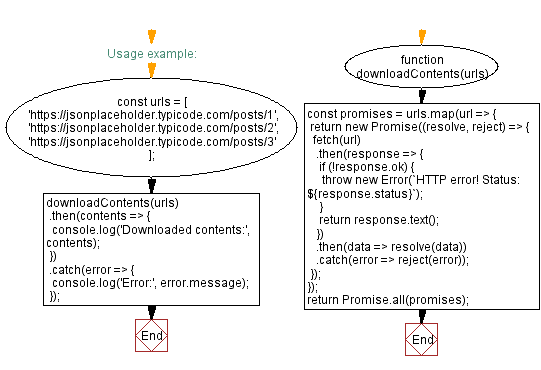
Live Demo:
See the Pen javascript-asynchronous-exercise-4 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that accepts an array of URLs and downloads their content in parallel using Promise.all, returning an array of responses.
- Write a JavaScript function that performs parallel HTTP GET requests on multiple URLs and filters out responses with errors, using Promises.
- Write a JavaScript function that concurrently fetches data from an array of URLs and maps each response to JSON using Promise.all.
- Write a JavaScript function that handles parallel downloads from multiple URLs, ensuring that a failure in one does not stop the others from completing.
Improve this sample solution and post your code through Disqus.
Asynchronous Exercises Previous: JavaScript HTTP GET Request with Promise.
Asynchronous Exercises Next: Sequential Asynchronous Operations in JavaScript using Promises and async/await.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.