Sequential Asynchronous Operations in JavaScript using Promises and async/await
JavaScript Asynchronous: Exercise-5 with Solution
Write a JavaScript program that implements a function that performs a series of asynchronous operations in sequence using Promises and 'async/await'.
Sample Solution:
JavaScript Code:
function asyncOperation1() {
return new Promise((resolve) => {
setTimeout(() => {
console.log('Asynchronous Operation 1');
resolve();
}, 1000);
});
}
function asyncOperation2() {
return new Promise((resolve) => {
setTimeout(() => {
console.log('Asynchronous Operation 2');
resolve();
}, 2000);
});
}
function asyncOperation3() {
return new Promise((resolve) => {
setTimeout(() => {
console.log('Asynchronous Operation 3');
resolve();
}, 1500);
});
}
async function performOperations() {
try {
await asyncOperation1();
await asyncOperation2();
await asyncOperation3();
console.log('All operations completed');
} catch (error) {
console.log('Error:', error.message);
}
}
performOperations();
Output:
"Asynchronous Operation 1" "Asynchronous Operation 2" "Asynchronous Operation 3" "All operations completed"
Note: Executed on JS Bin
Explanation:
In the above exercise -
- First we define three functions: asyncOperation1, asyncOperation2, and asyncOperation3. Each function returns a “Promise” that resolves after a certain delay using setTimeout. Inside the setTimeout callback, a console log is generated to simulate asynchronous operation.
- The "performOperations()" function is declared as an async function. This allows us to use the await keyword inside it to pause execution until each asynchronous operation completes.
- Inside the "performOperations()" method, the asynchronous operations are executed sequentially using await. The await keyword is used before each function call to pause execution until the respective "Promise" resolves. This ensures that each operation completes before moving on to the next one.
- If any error occurs during asynchronous operations, it will be caught in the catch block, where the error message is logged.
- After all the tasks have been completed, the program logs "All operations completed".
Flowchart:
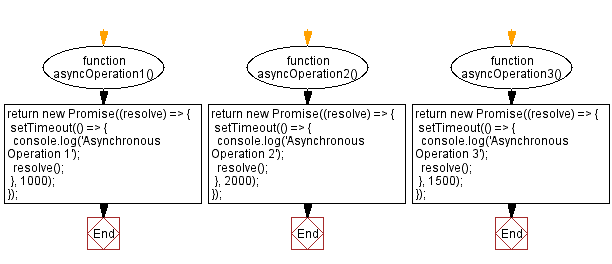
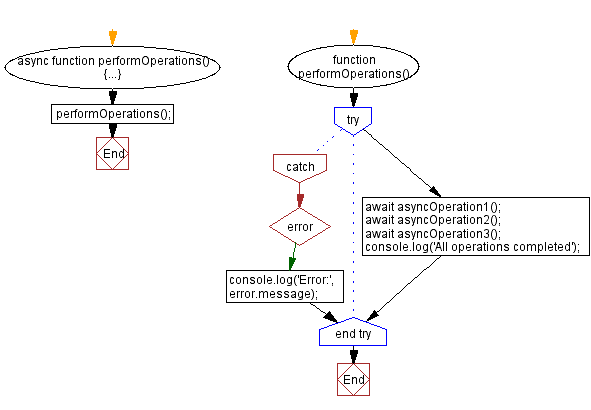
Live Demo:
See the Pen javascript-asynchronous-exercise-5 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Asynchronous Exercises Previous: Parallel URL Content Download with JavaScript Promises.
Asynchronous Exercises Next: Concurrent Data Fetching from Multiple APIs using Promises and Promise.all().
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics