Concurrent Data Fetching from Multiple APIs using Promises and Promise.all()
JavaScript Asynchronous: Exercise-6 with Solution
Concurrent API Fetch with Promise.all
Write a JavaScript function that fetches data from multiple APIs concurrently and returns a combined result using Promises and 'Promise.all()'.
Sample Solution:
JavaScript Code:
function fetchDataFromAPI(url) {
return fetch(url)
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
});
}
function fetchMultipleAPIs(apiUrls) {
const promises = apiUrls.map(url => fetchDataFromAPI(url));
return Promise.all(promises);
}
// Example usage:
const apiUrls = [
'https://jsonplaceholder.typicode.com/posts/4',
'https://jsonplaceholder.typicode.com/posts/5',
'https://jsonplaceholder.typicode.com/posts/6'
];
fetchMultipleAPIs(apiUrls)
.then(results => {
console.log('Combined Results:', results);
})
.catch(error => {
console.log('Error:', error.message);
});
Output:
"Combined Results:" [[object Object] { body: "ullam et saepe reiciendis voluptatem adipisci sit amet autem assumenda provident rerum culpa quis hic commodi nesciunt rem tenetur doloremque ipsam iure quis sunt voluptatem rerum illo velit", id: 4, title: "eum et est occaecati", userId: 1 }, [object Object] { body: "repudiandae veniam quaerat sunt sed alias aut fugiat sit autem sed est voluptatem omnis possimus esse voluptatibus quis est aut tenetur dolor neque", id: 5, title: "nesciunt quas odio", userId: 1 }, [object Object] { body: "ut aspernatur corporis harum nihil quis provident sequi mollitia nobis aliquid molestiae perspiciatis et ea nemo ab reprehenderit accusantium quas voluptate dolores velit et doloremque molestiae", id: 6, title: "dolorem eum magni eos aperiam quia", userId: 1 }]
Note: Executed on JS Bin
Explanation:
In the above exercise -
The "fetchMultipleAPIs()" function takes an array of API URLs as a parameter. It maps over the URLs and creates an array of Promises by calling fetchDataFromAPI for each URL.
Promise.all() is used to wait for all the Promises in the array to resolve. It returns a new Promise that resolves with an array of the resolved values from each Promise.
In the example usage, an array of API URLs is provided, and fetchMultipleAPIs is called with that array. The resulting Promise is then handled with .then() to log the combined results or with .catch() to handle any errors that occur during the fetching process.
Flowchart:
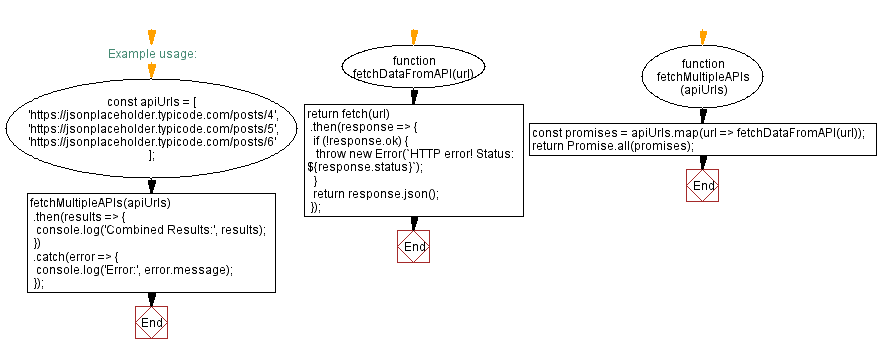
Live Demo:
See the Pen javascript-asynchronous-exercise-6 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that concurrently fetches data from multiple APIs using Promise.all and aggregates the results into a single array.
- Write a JavaScript function that performs concurrent fetch requests and filters out any responses that fail, using Promise.all and error handling.
- Write a JavaScript function that uses Promise.all to execute several API calls in parallel, then transforms the combined responses before returning them.
- Write a JavaScript function that concurrently requests data from different endpoints, and returns a consolidated object with the results mapped by API name.
Improve this sample solution and post your code through Disqus.
Asynchronous Exercises Previous: Sequential Asynchronous Operations in JavaScript using Promises and async/await.
Asynchronous Exercises Next: JavaScript Function for Retrying API Requests with Automatic Retry.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.