JavaScript Function for Retrying API Requests with Automatic Retry
JavaScript Asynchronous: Exercise-7 with Solution
Write a JavaScript function that fetches data from an API and retries the request a specified number of times if it fails.
Sample Solution:
JavaScript Code:
function fetchDataWithRetry(url, maxRetries) {
return new Promise((resolve, reject) => {
let retries = 0;
const fetchData = () => {
fetch(url)
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.then(data => resolve(data))
.catch(error => {
retries++;
if (retries <= maxRetries) {
console.log(`Request failed. Retrying (${retries}/${maxRetries})...`);
fetchData();
} else {
reject(new Error(`Failed after ${maxRetries} retries. Error: ${error.message}`));
}
});
};
fetchData();
});
}
// Usage example:
//const apiUrl = 'https://jsonplaceholder.typicode.com/posts3';
const apiUrl = 'https://jsonplaceholder.typicode.com/posts';
console.log("URL-> ",apiUrl);
const maxRetries = 3;
fetchDataWithRetry(apiUrl, maxRetries)
.then(data => {
console.log('Fetched data:', data);
})
.catch(error => {
console.log('Error:', error.message);
});
Output:
"URL-> " "https://jsonplaceholder.typicode.com/posts3" "Request failed. Retrying (1/3)..." "Request failed. Retrying (2/3)..." "Request failed. Retrying (3/3)..." "Error:" "Failed after 3 retries. Error: HTTP error! Status: 404"
"URL-> " "https://jsonplaceholder.typicode.com/posts" "Fetched data:" [[object Object] { body: "quia et suscipit suscipit recusandae consequuntur expedita et cum reprehenderit molestiae ut ut quas totam nostrum rerum est autem sunt rem eveniet architecto", id: 1, title: "sunt aut facere repellat provident occaecati excepturi optio reprehenderit", userId: 1 }, [object Object] { body: "est rerum tempore vitae sequi sint nihil reprehenderit dolor beatae ea dolores neque fugiat blanditiis voluptate porro vel nihil molestiae ut reiciendis qui aperiam non debitis possimus qui neque nisi nulla", id: 2, title: "qui est esse", userId: 1 }, [object Object] { body: "et iusto sed quo iure voluptatem occaecati omnis eligendi aut ad voluptatem doloribus vel accusantium quis pariatur molestiae porro eius odio et labore et velit aut", ………………………….. ………………………….. id: 27, title: "quasi id et eos tenetur aut quo autem", userId: 3 }, [object Object] { body: "non et quaerat ex quae ad maiores maiores recusandae totam aut blanditiis mollitia quas illo ………………………….. ………………………….. id: 99, title: "temporibus sit alias delectus eligendi possimus magni", userId: 10 }, [object Object] { body: "cupiditate quo est a modi nesciunt soluta ipsa voluptas error itaque dicta in autem qui minus magnam et distinctio eum accusamus ratione error aut", id: 100, title: "at nam consequatur ea labore ea harum", userId: 10 }]
Note: Executed on JS Bin
Explanation:
In the above exercise -
- The "fetchDataWithRetry()" function accepts two parameters: url specifies the API endpoint to fetch data from, and maxRetries indicates the maximum number of retries if the request fails.
- Inside the function, a counter 'retries' is initialized to keep track of the number of retries." The core logic is encapsulated in the "fetchData()" function, which performs the fetch request and handles success and failure cases.
- If the request is successful (response.ok), the data is resolved using resolve(data). Otherwise, if the request fails, the function checks if the number of retries is less than or equal to the 'maxRetries'. If so, it logs a retry message and recursively calls "fetchData()" again to retry the request. If the maximum number of retries is reached, it rejects the Promise with an error.
- In the usage example, the apiUrl specifies the API endpoint, and 'maxRetries' is set to 3. The function "fetchDataWithRetry()" is called, and the retrieved data or error is logged accordingly.
Flowchart:
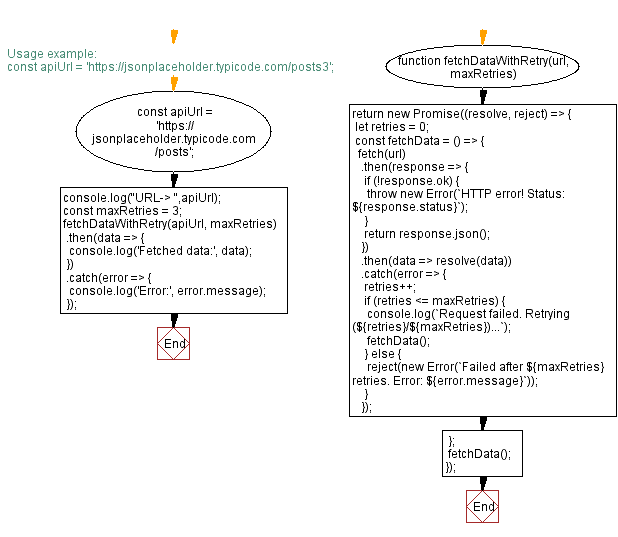
Live Demo:
See the Pen javascript-asynchronous-exercise-7 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Asynchronous Exercises Previous: Concurrent Data Fetching from Multiple APIs using Promises and Promise.all().
Asynchronous Exercises Next: Repeatedly executing a function at fixed intervals using setInterval().
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics