JavaScript Program: Repeatedly executing a function at fixed intervals using setInterval()
JavaScript Asynchronous: Exercise-8 with Solution
Write a JavaScript program to implement a function that executes a given function repeatedly at a fixed interval using 'setInterval()'.
Sample Solution:
JavaScript Code:
function repeat_Function(fn, interval) {
// Execute the function immediately
fn();
// Set up the interval to execute the function repeatedly
const intervalId = setInterval(fn, interval);
// Return a function to stop the execution
return function stopExecution() {
clearInterval(intervalId);
console.log('Execution stopped.');
};
}
// Usage example:
const intervalMs = 1000; // 1 second
// Define the function to be repeated
function printMessage() {
console.log('Executing the function...');
}
// Start the repeated execution
const stopExecution = repeat_Function(printMessage, intervalMs);
// Stop the execution after 4 seconds
setTimeout(() => {
stopExecution();
}, 4000);
Output:
"Executing the function..." "Executing the function..." "Executing the function..." "Executing the function..." "Executing the function..." "Execution stopped."
Note: Executed on JS Bin
Explanation:
In the above exercise -
- The "repeatFunction()" function takes two parameters: fn represents the function to be executed repeatedly, and interval specifies the time interval (in milliseconds) between each execution.
- Inside repeatFunction, the provided function fn is executed immediately to ensure it runs before starting the interval.
- 'setInterval' is used to set up repeated execution. The returned intervalId is stored to clear the interval if needed.
- The function returns another function (stopExecution) that stops execution when called. This function clears the interval using clearInterval and logs a message indicating that execution has stopped.
In the example, a time interval of 1 second (1000 milliseconds) is specified. The "printMessage()" function is defined to log a message, and repeatFunction is called to start repeated execution. After 4 seconds (4000 milliseconds), the stopExecution function is invoked to stop execution.
Flowchart:
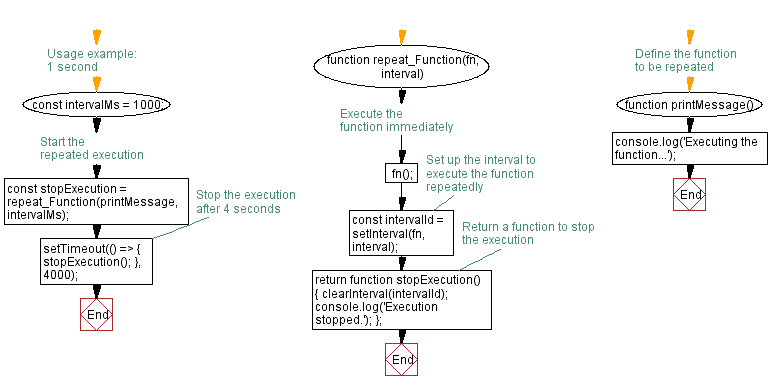
Live Demo:
See the Pen javascript-asynchronous-exercise-8 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Asynchronous Exercises Previous: JavaScript Function for Retrying API Requests with Automatic Retry.
Asynchronous Exercises Next: Fetching data from API with request timeout.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics