JavaScript Function: Fetching data from API with request timeout
JavaScript Asynchronous: Exercise-9 with Solution
API Fetch with Timeout Cancellation
Write a JavaScript function that fetches data from an API and cancels the request if it takes longer than a specified time.
Sample Solution:
JavaScript Code:
function fetchDataWithTimeout(url, timeout) {
const controller = new AbortController();
const { signal } = controller;
const timeoutId = setTimeout(() => {
controller.abort();
}, timeout);
return fetch(url, { signal })
.then(response => {
clearTimeout(timeoutId);
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.catch(error => {
if (error.name === 'AbortError') {
throw new Error('Request timed out.');
} else {
throw error;
}
});
}
// Usage example:
const url = 'https://jsonplaceholder.typicode.com/posts/1';
const timeoutMs = 5000;
//const timeoutMs = 50;
console.log("Timeout milliseconds: "+timeoutMs)
fetchDataWithTimeout(url, timeoutMs)
.then(data => {
console.log('Data:', data);
})
.catch(error => {
console.log('Error:', error.message);
});
Output:
"Timeout milliseconds: 5000" "Data:" [object Object] { body: "quia et suscipit suscipit recusandae consequuntur expedita et cum reprehenderit molestiae ut ut quas totam nostrum rerum est autem sunt rem eveniet architecto", id: 1, title: "sunt aut facere repellat provident occaecati excepturi optio reprehenderit", userId: 1 }
"Timeout milliseconds: 50" "Error:" "Request timed out."
Note: Executed on JS Bin
Explanation:
In the above exercise -
- The function "fetchDataWithTimeout()" takes two parameters: url (the URL to fetch data from) and timeout (the maximum time in milliseconds allowed for the request).
- It creates an 'AbortController' instance and obtains its associated signal. The 'AbortController' cancels the request if it exceeds the specified timeout.
- A timeout is set using setTimeout, which calls controller.abort() when the specified timeout duration elapses. This cancels the request by aborting it.
- The function uses the fetch API to make the actual request, passing the signal to the options object to associate it with the request.
- If the response is successful (response.ok is true), the function clears the timeout using clearTimeout(timeoutId) and parses the response data as JSON using response.json().
- If the response is not successful, an error is thrown with a message indicating HTTP error status.
- If an error occurs during the request, the function checks if the error name is 'AbortError', which indicates that the request was aborted due to a timeout. In this case, it throws an error with the message "Request timed out." Otherwise, it rethrows the original error.
- The function returns a Promise that resolves with the parsed response data or rejects with an error.
In the usage example, the "fetchDataWithTimeout()" function is called with a sample URL and a timeout of 5000 milliseconds and 50 milliseconds. The fetched data is logged if the request is successful, and any errors are caught and logged.
Flowchart:
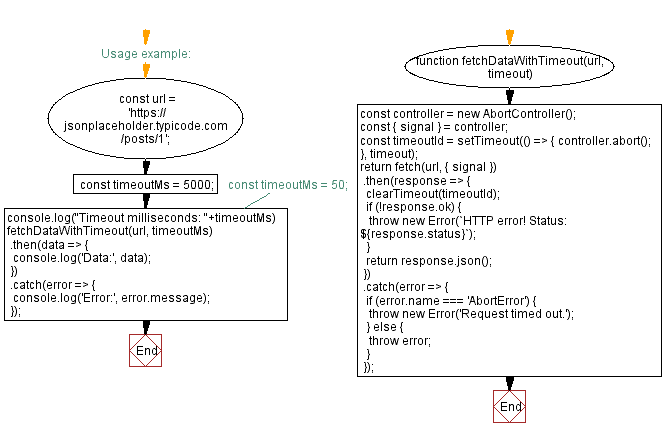
Live Demo:
See the Pen javascript-asynchronous-exercise-9 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that performs an API fetch and cancels the request using an AbortController if the response takes longer than a specified timeout.
- Write a JavaScript function that implements a timeout mechanism with Promise.race, cancelling the fetch request if it exceeds a set duration.
- Write a JavaScript function that performs an API fetch with a timeout, aborts the request if it delays too long, and returns a custom error message.
- Write a JavaScript function that combines fetch with a timeout cancellation strategy, retrying the fetch if it fails due to a timeout.
Go to:
PREV : Repeated Execution with setInterval.
NEXT : JavaScript OOP Exercises Home.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.