JavaScript conversion: Callback to Promise | Transforming asynchronous functions
JavaScript Asynchronous: Exercise-2 with Solution
Callback to Promise Conversion
Write a JavaScript program that converts a callback-based function to a Promise-based function.
Promise:
The Promise object represents the eventual completion (or failure) of an asynchronous operation and its resulting value.
A Promise is a proxy for a value not necessarily known when the promise is created. It allows you to associate handlers with an asynchronous action's eventual success value or failure reason. This lets asynchronous methods return values like synchronous methods: instead of immediately returning the final value, the asynchronous method returns a promise to supply the value at some point in the future.
A Promise is in one of these states:
- pending: initial state, neither fulfilled nor rejected.
- fulfilled: meaning that the operation was completed successfully.
- rejected: meaning that the operation failed.
Sample Solution:
JavaScript Code:
function callback_BasedFunction(arg1, arg2, callback) {
// Perform asynchronous operations
// Call the callback with the result or error
setTimeout(() => {
const result = arg1 + arg2;
if (result % 2 !== 0) {
callback(null, result);
} else {
callback(new Error('Result is not odd!'), null);
}
}, 1000);
}
function promisifiedFunction(arg1, arg2) {
return new Promise((resolve, reject) => {
callback_BasedFunction(arg1, arg2, (error, result) => {
if (error) {
reject(error); // Reject the Promise with the error
} else {
resolve(result); // Resolve the Promise with the result
}
});
});
}
// Usage example:
promisifiedFunction(2, 3)
.then(result => {
console.log('Result:', result);
})
.catch(error => {
console.log('Error:', error.message);
});
promisifiedFunction(2, 4)
.then(result => {
console.log('Result:', result);
})
.catch(error => {
console.log('Error:', error.message);
});
Output:
"Result:" 5 "Error:" "Result is not odd!"
Note: Executed on JS Bin
Explanation:
In the above exercise,
The "callback_BasedFunction()" function takes two arguments arg1 and arg2, and a callback function "callback". Inside this function, it performs some asynchronous operations using setTimeout to simulate a delay. After the delay, it calculates the sum of arg1 and arg2. If the result is not an odd number, it calls the callback with an error (new Error('Result is not odd!')) and a null result; otherwise, it calls the callback with null error and the calculated result.
The "promisifiedFunction()" function is a wrapper function that converts "callback_BasedFunction()" function into a Promise-based function. It takes the same arguments arg1 and arg2. Inside the Promise's executor function, it calls "callback_BasedFunction()" function with the provided arguments and a callback. Depending on the result, the Promise is resolved by calling resolve(result) or rejected by calling reject(error).
Finally in the usage examples, the first one passes arguments 2 and 3, which will result in an odd number, so the Promise will be resolved. The second invocation passes arguments 2 and 4, which will result in an even number, so the Promise will be rejected.
Flowchart:
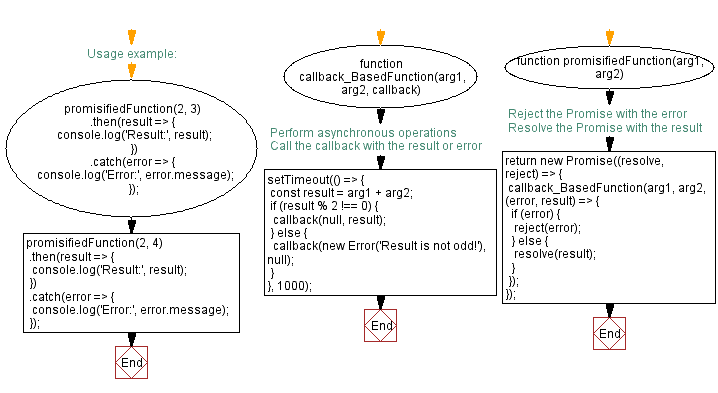
Live Demo:
See the Pen javascript-asynchronous-exercise-2 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Asynchronous Exercises Previous: Invoke Callback after a specific second.
Asynchronous Exercises Next: JavaScript HTTP GET Request with Promise.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/asynchronous/javascript-asynchronous-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics