JavaScript delayed callback function - Invoke Callback after a specific second
JavaScript Asynchronous: Exercise-1 with Solution
Delayed Callback Execution
Write a JavaScript function that takes a callback and invokes it after a delay of 2 second.
Sample Solution:
JavaScript Code:
function invokeAfterDelay(callback) {
setTimeout(callback, 2000); // 2000 milliseconds = 2 second
}
function display_message() {
console.log('Hello!');
}
invokeAfterDelay(display_message); // Invokes the sayHello function after a 1-second delay
Output:
"Hello!"
Note: Executed on JS Bin
Explanation:
In the above exercise JavaScript function "invokeAfterDelay()" takes a callback and invokes it after a delay of 2 second (2000 milliseconds) using the "setTimeout()" function.
In the above function, the "display_message()" function will be invoked after a delay of 2 seconds.
Flowchart:
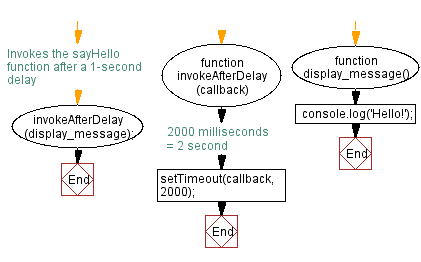
Live Demo:
See the Pen javascript-asynchronous-exercise-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that accepts a callback and a custom delay value, then executes the callback after the specified delay, logging the start and end times.
- Write a JavaScript function that schedules a callback to run after 2 seconds, but cancels the execution if the function is called again within that period.
- Write a JavaScript function that simulates delayed callback execution using recursive setTimeout calls, triggering the callback after a cumulative delay.
- Write a JavaScript function that accepts a callback and a delay, ensuring the callback is invoked only once even if multiple calls are made before the delay expires.
Improve this sample solution and post your code through Disqus.
Asynchronous Exercises Previous: JavaScript Asynchronous Exercises Home.
Asynchronous Exercises Next: Callback to Promise | Transforming asynchronous functions.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.