JavaScript Function: Custom Error on an empty string
JavaScript Error Handling: Exercise-6 with Solution
Write a JavaScript function that takes a string as a parameter and throws a custom 'Error' if the string is empty.
Sample Solution:
JavaScript Code:
// Define a function named validate_String_Not_Empty that takes one parameter: str
function validate_String_Not_Empty(str) {
// Check if the length of the string is equal to 0
if (str.length === 0) {
// If the string is empty, throw an error indicating that the string is empty
throw new Error('Sorry, the string is empty!');
}
}
// Example:
try {
// Create a string string1 with value 'Hello, World!'
const string1 = 'Hello, World!';
// Call the validate_String_Not_Empty function with string1 as an argument, which is a valid non-empty string
validate_String_Not_Empty(string1); // Valid non-empty string
// Create an empty string string2
const string2 = '';
// Call the validate_String_Not_Empty function with string2 as an argument, which is an empty string and will throw an error
validate_String_Not_Empty(string2); // Throws an error
} catch (error) {
// Catch and log any error that occurs during execution
console.log('Error:', error.message);
}
Output:
"Error:" "Sorry, the string is empty!"
Note: Executed on JS Bin
Explanation:
In the "validate_String_Not_Empty()" function, we check the string length using the length property. If the length is 0, we throw an 'Error' with the custom message 'Sorry, the string is empty!'.
Next, we call the "validate_String_Not_Empty()" function twice. The first call with string1 containing 'Hello, World!' is a valid non-empty string, so no error is thrown. The second call with string2 containing an empty string throws an error, which is caught by the catch block. In the catch block, we log the custom error message using error.message.
Flowchart:
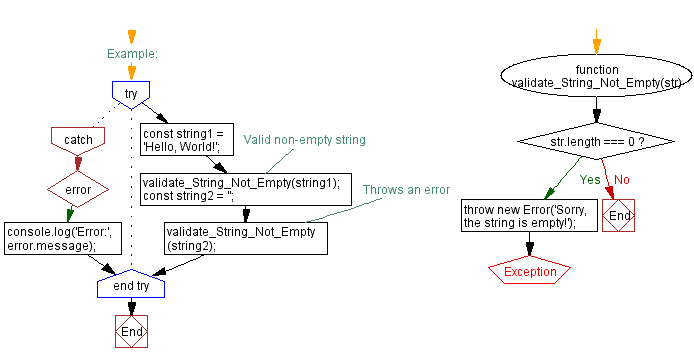
Live Demo:
See the Pen javascript-error-handling-exercise-6 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Error Handling Exercises Previous: Custom Error on an empty string.
Error Handling Exercises Next: Catching and handling RangeError with Try-Catch.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics