JavaScript Program: Catching and handling RangeError with Try-Catch
JavaScript Error Handling: Exercise-7 with Solution
Write a JavaScript program that uses a try-catch block to catch and handle a 'RangeError' when accessing an array with an invalid index.
Sample Solution:
JavaScript Code:
// Define a function named access_Array_Element that takes two parameters: array and index
function access_Array_Element(array, index) {
// Attempt to access the element at the given index in the array
try {
// Access the element at the specified index in the array
const element = array[index];
// Log the accessed element to the console
console.log('Accessed element:', element);
} catch (error) {
// If an error occurs during execution
// Check if the error is a RangeError
if (error instanceof RangeError) {
// If the error is a RangeError, log a message indicating that the index is invalid
console.log('Error: Invalid index. Please provide a valid index within the array bounds.');
} else {
// If the error is not a RangeError, re-throw the error to propagate it further
throw error; // re-throw the error if it's not a RangeError
}
}
}
// Example
// Create an array named nums with some elements
const nums = [1, 2, 3, 4, 5];
// Call the access_Array_Element function with nums and index 1, which is a valid index
access_Array_Element(nums, 1); // Valid index
// Call the access_Array_Element function with nums and index 5, which is an invalid index
access_Array_Element(nums, 5); // Invalid index
Output:
"Accessed element:" 2 "Accessed element:" undefined
Note: Executed on JS Bin
Explanation:
In the above exercise, the "access_Array_Element()" function uses a try-catch block to attempt to access an element in the array using the given index. If an error occurs, we check if it is an instance of RangeError (which is the specific error thrown for an invalid array index). If it is a RangeError, we handle it by logging a custom error message. Otherwise, we re-throw the error to be caught by an outer catch block, if any.
Next, we create an array 'nums' with elements [1, 2, 3, 4, 5]. We call the "access_Array_Element()" function twice, passing different indices. The first call with index 1 is valid and outputs the accessed element 2. The second call with index 5 is invalid and throws a RangeError, which is caught by the catch block, and the custom error message is displayed.
Flowchart:
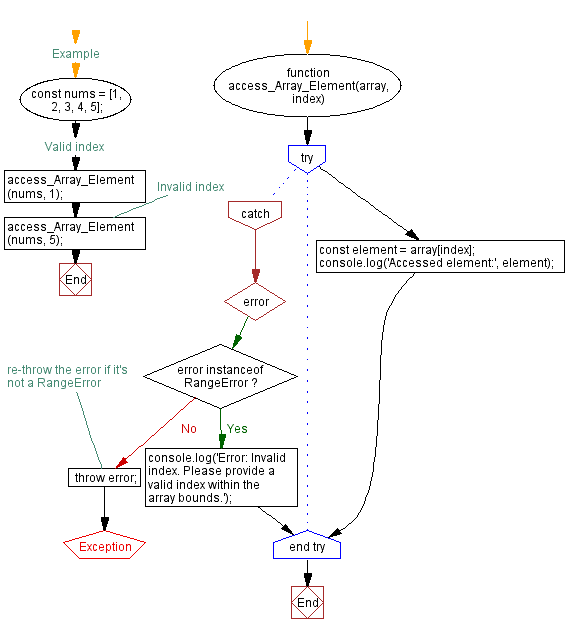
Live Demo:
See the Pen javascript-error-handling-exercise-7 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Error Handling Exercises Previous: Custom Error on an empty string.
Error Handling Exercises Next: Handling different types of errors with multiple catch blocks.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics