JavaScript Program: Handling different types of errors with multiple catch blocks
JavaScript Error Handling: Exercise-8 with Solution
Multiple Catch Blocks
Write a JavaScript program that shows the use of multiple catch blocks to handle different types of errors separately.
Sample Solution:
JavaScript Code:
// Define a function named divide_Numbers that takes two parameters: x and y
function divide_Numbers(x, y) {
// Attempt to perform division operation
try {
// Check if both x and y are numbers
if (typeof x !== 'number' || typeof y !== 'number') {
// If either x or y is not a number, throw a TypeError
throw new TypeError('Invalid arguments. Both arguments should be numbers.');
}
// Check if y is zero
if (y === 0) {
// If y is zero, throw an Error indicating division by zero is not allowed
throw new Error('Invalid divisor. Cannot divide by zero.');
}
// Perform division operation
const result = x / y;
// Log the result of division to the console
console.log('Result:', result);
} catch (error) {
// If an error occurs during execution
// Check if the error is a TypeError
if (error instanceof TypeError) {
// If the error is a TypeError, log the error message with 'TypeError' prefix
console.log('TypeError:', error.message);
} else {
// If the error is not a TypeError, log the error message with 'Error' prefix
console.log('Error:', error.message);
}
}
}
// Example:
// Call the divide_Numbers function with 20 and '4', where '4' is a string instead of a number
divide_Numbers(20, '4'); // Invalid divisor
// Call the divide_Numbers function with 20 and 4, which is a valid division operation
divide_Numbers(20, 4); // Valid division
// Call the divide_Numbers function with 20 and 0, which results in division by zero
divide_Numbers(20, 0); // Division by zero
Output:
TypeError:" "Invalid arguments. Both arguments should be numbers." "Result:" 5 "Error:" "Invalid divisor. Cannot divide by zero."
Note: Executed on JS Bin
Explanation:
In the above exercise,
- The "divide_Numbers()" function takes two parameters x and y. Inside the function, we have a try block where we attempt to perform a division operation using the provided arguments.
- Within the try block, there are two if conditions to validate the arguments. The first condition checks if x or y is not a number using the typeof operator. If either of them is not a number, we throw a TypeError using the throw statement with a custom error message.
- The second condition checks if the divisor y is equal to zero. If it is, we throw a generic Error using the throw statement with a custom error message.
- If both conditions are met, we proceed with the division operation and store the result in the result variable. We then log the result to the console.
- If any error occurs within the try block, it is caught in the catch block. The catch block takes an error parameter representing the thrown error object. Inside the catch block, we use an if-else statement to determine the type of error.
- If the error is an instance of TypeError, we log a message with the prefix 'TypeError:' followed by the error message. Otherwise, for other types of errors, we log a message with the prefix 'Error:' followed by the error message.
Next the "divide_Numbers()" function is called three times with different arguments. The first call with 20 and '4' results in an invalid divisor since the second argument is not a number. The second call with 20 and 4 is a valid division and prints the result. The third call with 20 and 0 throws an error due to division by zero.
Flowchart:
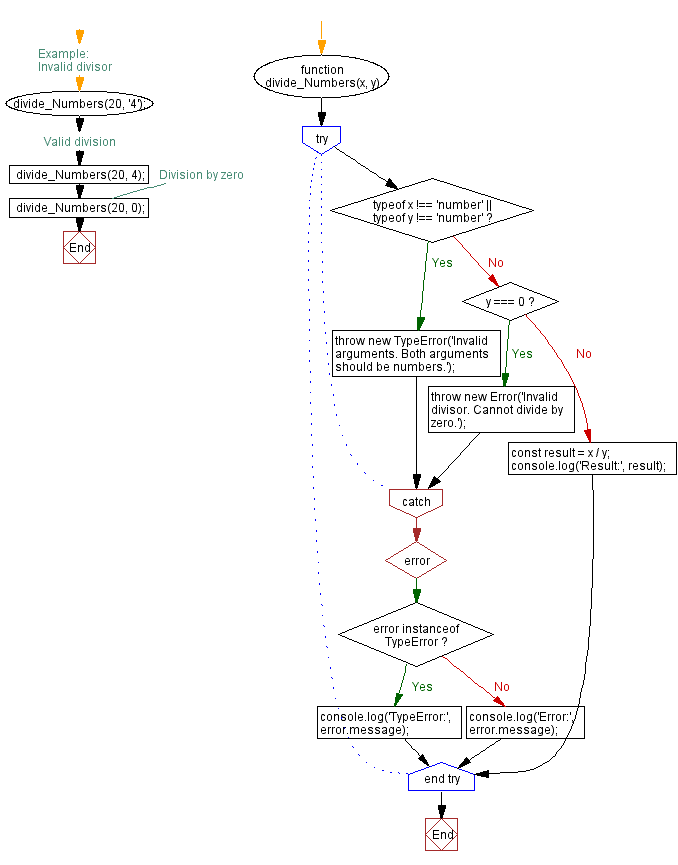
Live Demo:
See the Pen javascript-error-handling-exercise-8 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that uses nested try-catch blocks to separately handle TypeError and ReferenceError raised in the same code segment.
- Write a JavaScript function that triggers different error types in a loop and distinguishes them in a single catch block by inspecting the error type.
- Write a JavaScript function that simulates multiple errors in sequence and demonstrates custom handling for each error type within one try-catch-finally structure.
- Write a JavaScript function that evaluates various expressions and uses conditional logic in the catch block to mimic multiple catch clauses.
Improve this sample solution and post your code through Disqus.
Error Handling Exercises Previous: Catching and handling RangeError with Try-Catch.
Error Handling Exercises Next: Handling URIError with Try-Catch block for invalid URI decoding.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.