JavaScript Function: Custom Error on an empty array
JavaScript Error Handling: Exercise-5 with Solution
Write a JavaScript function that takes an array as a parameter and throws a custom 'Error' if the array is empty.
Sample Solution:
JavaScript Code:
// Define a function named validateArrayNotEmpty that takes one parameter: arr
function validateArrayNotEmpty(arr) {
// Check if the length of the array is equal to 0
if (arr.length === 0) {
// If the array is empty, throw an error indicating that the array is empty
throw new Error('Array is empty!');
}
}
// Example usage:
try {
// Create an array nums1 with elements [1, 2, 3, 4]
const nums1 = [1, 2, 3, 4];
// Call the validateArrayNotEmpty function with nums1 as an argument, which is a valid non-empty array
validateArrayNotEmpty(nums1); // Valid non-empty array
// Create an empty array nums2
const nums2 = [];
// Call the validateArrayNotEmpty function with nums2 as an argument, which is an empty array and will throw an error
validateArrayNotEmpty(nums2); // Throws an error
} catch (error) {
// Catch and log any error that occurs during execution
console.log('Error:', error.message);
}
Output:
"Error:" "Array is empty!"
Note: Executed on JS Bin
Explanation:
In the above example -
The "validateArrayNotEmpty()" function checks the array length using the length property. If the length is 0, we throw an 'Error' with the custom message 'Array is empty!'.
In the example usage, we call the "validateArrayNotEmpty()" function twice. The first call with nums1 containing [1, 2, 3, 4] is a valid non-empty array, so no error is thrown. The second call with nums2 containing an empty array [] throws an error, which is caught by the catch block. In the catch block, we log the custom error message using error.message.
Flowchart:
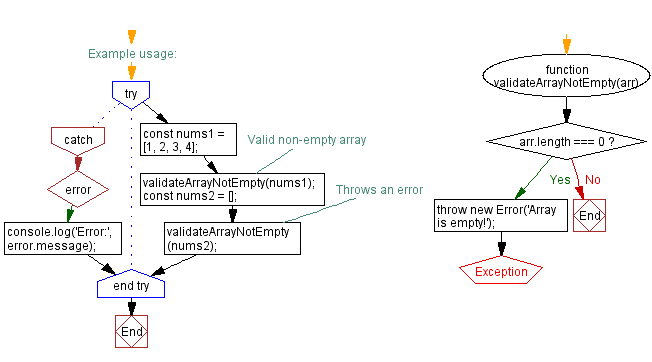
Live Demo:
See the Pen javascript-error-handling-exercise-5 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Error Handling Exercises Previous: Custom Error on a negative number.
Error Handling Exercises Next: Custom Error on an empty string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics