JavaScript Function: Custom Error on a negative number
JavaScript Error Handling: Exercise-4 with Solution
Throw Error for Negative Number
Write a JavaScript function that takes a number as a parameter and throws a custom 'Error' if the number is negative.
Sample Solution:
JavaScript Code:
// Define a function named validate_Positive_Number that takes one parameter: n
function validate_Positive_Number(n) {
// Check if n is less than 0
if (n < 0) {
// If n is negative, throw an error indicating negative numbers are not allowed
throw new Error('Error: Negative numbers are not allowed.');
}
// If n is not negative, return n
return n;
}
// Call the validate_Positive_Number function with argument 3 and log the result to the console
console.log(validate_Positive_Number(3));
// Call the validate_Positive_Number function with argument -5, which will throw an error, and handle it
console.log(validate_Positive_Number(-5));
Output:
3 "error" "Error: Error: Negative numbers are not allowed.
Note: Executed on JS Bin
Explanation:
In the above exercise,
The "validatePositiveNumber()" function checks if a number "n" is less than zero using the comparison operator <. If it is, we throw a custom Error with the message 'Error: Negative numbers are not allowed.' This ensures that the function will not proceed with further operations if a negative number is passed.
If the number is not negative, the function returns the number itself.
Flowchart:
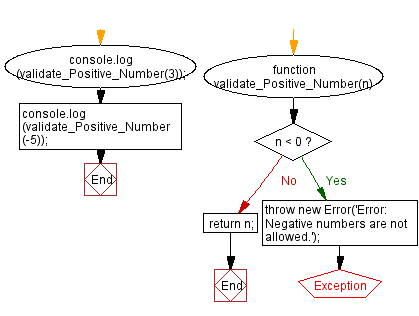
Live Demo:
See the Pen javascript-error-handling-exercise-4 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that throws a custom Error when a negative number is passed, with support for BigInt inputs.
- Write a JavaScript function that evaluates an array of numbers and throws a composite Error if one or more values are negative.
- Write a JavaScript function that calculates the square root of a number and throws an Error if the input is negative.
- Write a JavaScript function that parses a numeric string and throws a custom Error when the parsed number is negative, addressing edge cases.
Go to:
PREV : Throw Error for Division by Zero.
NEXT : Throw Error for Empty Array.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.