JavaScript toggle switch: Interactive state change
JavaScript Event Handling: Exercise-7 with Solution
Toggle Switch Implementation
Write a JavaScript program to implement a toggle switch that changes its state when clicked.
Sample Solution:
HTML and JavaScript Code:
<!DOCTYPE html>
<html>
<head>
<style>
.toggle {
display: inline-block;
width: 60px;
height: 34px;
position: relative;
border-radius: 34px;
background-color: #ccd;
cursor: pointer;
transition: background-color 0.3s;
}
.toggle::before {
content: "";
position: absolute;
width: 24px;
height: 24px;
border-radius: 50%;
background-color: #fff;
top: 4px;
left: 4px;
transition: transform 0.3s;
}
.toggle.on {
background-color: #66bb6a;
}
.toggle.on::before {
transform: translateX(26px);
}
</style>
</head>
<body>
<div class="toggle"></div>
<script>
const toggle = document.querySelector('.toggle');
toggle.addEventListener('click', () => {
toggle.classList.toggle('on');
});
</script>
</body>
</html>
Output:
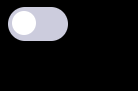
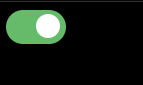
Note: Executed on JS Bin
Explanation:
In the above exercise,
We have a <div> element with the class toggle that represents a toggle switch. The switch has an initial state of "off" (default) defined by the .toggle class. When the switch is toggled on, the .on class is added to the toggle element, changing its appearance.
CSS styles define the toggle switch's appearance and behavior. The .toggle class sets the dimensions, position, border radius, and transition properties. The .toggle::before pseudo-element creates the circular handle of the switch. The .toggle.on class changes the background color of the switch when it is toggled on. The .toggle.on::before class transforms the handle to the "on" position.
The JavaScript code attaches a click event listener to the toggle element. When the toggle switch is clicked, the event listener toggles the .on class on the toggle element using the classList.toggle() method. This changes the state of the switch and triggers the corresponding CSS styles for the on or off state.
Flowchart:
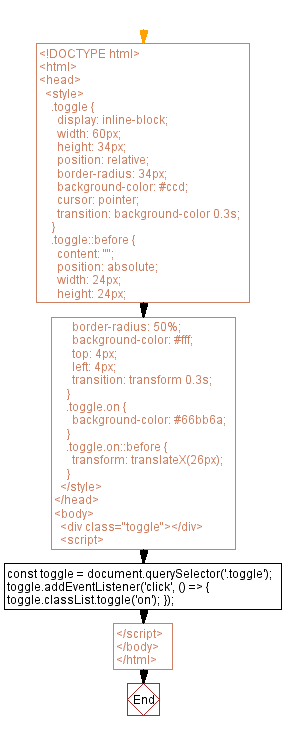
Live Demo:
See the Pen javascript-event-handling-exercise-7 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Event Handling Exercises Previous: JavaScript Drag-and-Drop: Reorder items in a list.
Event Handling Exercises Next: JavaScript progress bar: Dynamic task completion tracking.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/event/javascript-event-handling-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics