JavaScript progress bar: Dynamic task completion tracking
JavaScript Event Handling: Exercise-8 with Solution
Progress Bar Update
Write a JavaScript program to create a progress bar that updates its width based on task completion.
Sample Solution:
HTML and JavaScript Code:
<!DOCTYPE html>
<html>
<head>
<style>
.progress-bar {
width: 300px;
height: 20px;
background-color: #f0f0f0;
border-radius: 10px;
overflow: hidden;
}
.progress-bar-fill {
height: 100%;
background-color: #4caf50;
transition: width 0.3s;
}
</style>
</head>
<body>
<div class="progress-bar">
<div class="progress-bar-fill" id="progress"></div>
</div>
<script>
function updateProgress(progressPercentage) {
const progressBarFill = document.getElementById('progress');
progressBarFill.style.width = `${progressPercentage}%`;
}
// Example usage: updating progress to 75%
updateProgress(75);
</script>
</body>
</html>
Output:
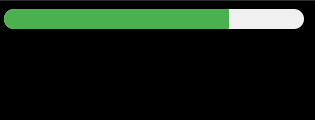
Note: Executed on JS Bin
Explanation:
In the above exercise,
- The HTML structure consists of a <div> element with a class of "progress-bar". This serves as the progress bar container. Inside this container, there is another <div> element with a class of "progress-bar-fill" and an id of "progress". This inner div represents the progress bar itself and will be dynamically updated to reflect progress.
- The CSS styles defined in the <style> block specify the progress bar appearance. The "progress-bar" class sets the width, height, background color, border radius, and overflow properties. The "progress-bar-fill" class sets the height and background color, and includes a transition effect to smoothly update the width property.
- The JavaScript code defines a function named "updateProgress" that takes a parameter called "progressPercentage". Inside the function, it retrieves the element with the id "progress" using document.getElementById('progress'). This element represents the progress bar fill.
- At the end of the code, there is an example usage of the "updateProgress" function, setting the progress to 75% by calling updateProgress(75).
Flowchart:
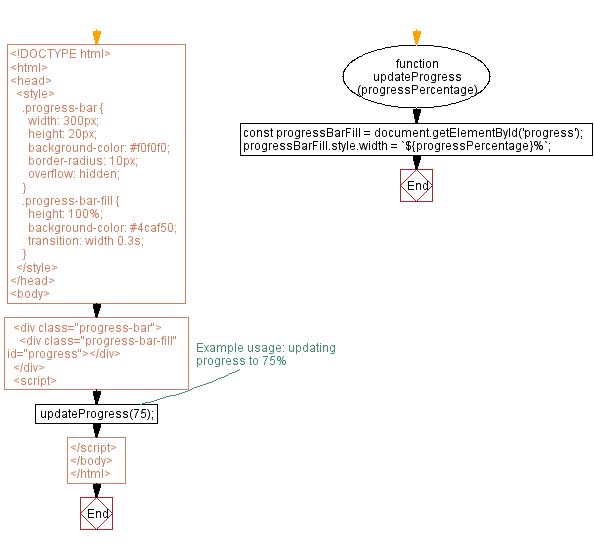
Live Demo:
See the Pen javascript-event-handling-exercise-8 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Event Handling Exercises Previous: JavaScript toggle switch: Interactive state change.
Event Handling Exercises Next: JavaScript Enter key detection: Listen for keydown events on text input.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/event/javascript-event-handling-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics