JavaScript Enter key detection: Listen for keydown events on text input
JavaScript Event Handling: Exercise-9 with Solution
Enter Key Detection in Input
Write a JavaScript program that adds a keydown event listener to a text input to detect when the "Enter key" is pressed.
Sample Solution:
HTML and JavaScript Code:
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<input type="text" id="textInput" placeholder="Press Enter...">
<script>
const textInput = document.getElementById('textInput');
textInput.addEventListener('keydown', (event) => {
if (event.key === 'Enter') {
console.log('Enter key pressed!');
// Perform desired actions here
}
});
</script>
</body>
</html>
Output:
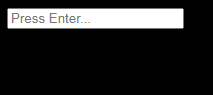
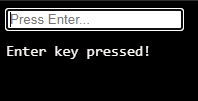
Note: Executed on JS Bin
Explanation:
In the above exercise -
- First we define an input element with the id "textInput". The JavaScript code retrieves this input element using document.getElementById('textInput') and assigns it to the textInput variable.
- Next we attach an event listener to the text input using the addEventListener method. We specify the event type as "keydown" to detect when any key is pressed while the text input has focus.
- Inside the event listener function, we check if the event.key property is equal to "Enter". If it is, we log a message to the console indicating that the Enter key was pressed.
Flowchart:
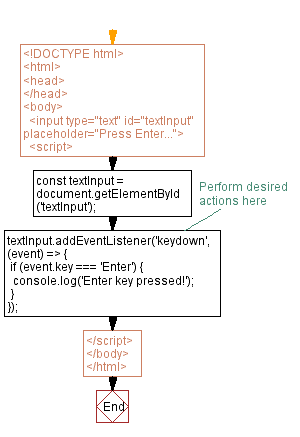
Live Demo:
See the Pen javascript-event-handling-exercise-9 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Event Handling Exercises Previous: JavaScript progress bar: Dynamic task completion tracking.
Event Handling Exercises Next: JavaScript double click event: Action on element.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/event/javascript-event-handling-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics