JavaScript double click event: Action on element
JavaScript Event Handling: Exercise-10 with Solution
Write a JavaScript function that listens for a double click on an element and performs a specific action.
Sample Solution:
HTML and JavaScript Code:
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<button id="myButton">Double click me!</button>
<script>
const myButton = document.getElementById('myButton');
myButton.addEventListener('dblclick', () => {
console.log('A double click has been performed!');
// Perform desired actions here
});
</script>
</body>
</html>
Output:
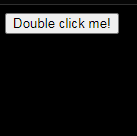
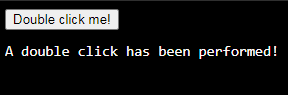
Note: Executed on JS Bin
Explanation:
In the above exercise,
- First, we define a button element with the id "myButton". The JavaScript code retrieves this button element using document.getElementById('myButton') and assigns it to the myButton variable.
- Next we attach an event listener to the button using the addEventListener method. We specify the event type as "dblclick" to detect double clicks on the button.
- Inside the event listener function, we log a message to the console indicating that the double click action was performed. You can replace the console.log statement with your desired action.
Flowchart:
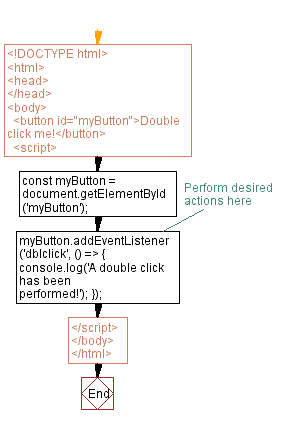
Live Demo:
See the Pen javascript-event-handling-exercise-10 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Event Handling Exercises Previous: JavaScript progress bar: Dynamic task completion tracking.
JavaScript functions Exercises Next: JavaScript functions Exercises Home.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics