JavaScript: Check if the given number falls within the given range
JavaScript fundamental (ES6 Syntax): Exercise-217 with Solution
Number in Range
Write a JavaScript program to check if the given number falls within the given range.
- Use arithmetic comparison to check if the given number is in the specified range.
- If the second argument, end, is not specified, the range is considered to be from 0 to start.
Sample Solution:
JavaScript Code:
// Define a function 'inRange' that checks if a number 'n' is within a specified range defined by 'start' and 'end' values
const inRange = (n, start, end = null) => {
// If 'end' is provided and 'start' is greater than 'end', swap their values
if (end && start > end) end = [start, (start = end)][0];
// If 'end' is not provided, check if 'n' is greater than or equal to 0 and less than 'start'
return end == null ? n >= 0 && n < start : n >= start && n < end;
};
// Test the 'inRange' function with sample inputs
console.log(inRange(3, 2, 5));
console.log(inRange(3, 4));
console.log(inRange(2, 3, 5));
console.log(inRange(3, 2));
Output:
true true false false
Flowchart:
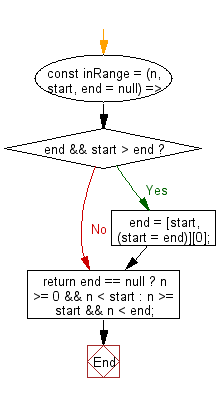
Live Demo:
See the Pen javascript-basic-exercise-217-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if a number falls within a given inclusive range and returns a boolean.
- Write a JavaScript function that validates a number against two boundary values and outputs a custom message.
- Write a JavaScript program that returns true if the input number is between a specified min and max, false otherwise.
- Write a JavaScript function that handles edge cases where the number is exactly equal to the boundaries.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get all indices of val in an array. If val never occurs, returns [].
Next: Write a JavaScript program to get the number of times a function executed per second.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.