JavaScript: Get the number of times a function executed per second
JavaScript fundamental (ES6 Syntax): Exercise-218 with Solution
Write a JavaScript program to get the number of times a function executed per second. HZ is the unit for hertz, the unit of frequency defined as one cycle per second.
- Use performance.now() to get the difference in milliseconds before and after the iteration loop to calculate the time elapsed executing the function iterations times.
- Return the number of cycles per second by converting milliseconds to seconds and dividing it by the time elapsed.
- Omit the second argument, iterations, to use the default of 100 iterations.
Sample Solution:
JavaScript Code:
// Define a function 'hz' to measure the performance (in Hertz) of a given function by running it for a specified number of iterations
const hz = (fn, iterations = 100) => {
// Record the current timestamp before executing the function
const before = performance.now();
// Execute the function 'iterations' times
for (let i = 0; i < iterations; i++) fn();
// Calculate the Hertz value by dividing the total number of iterations by the time taken to execute the function
return (1000 * iterations) / (performance.now() - before);
};
// Create an array 'numbers' containing 10,000 elements
const numbers = Array(10000)
.fill()
.map((_, i) => i);
// Define two functions 'sumReduce' and 'sumForLoop' to sum up the elements in the 'numbers' array using different methods
const sumReduce = () => numbers.reduce((acc, n) => acc + n, 0);
const sumForLoop = () => {
let sum = 0;
for (let i = 0; i < numbers.length; i++) sum += numbers[i];
return sum;
};
// Compare the performance of 'sumReduce' and 'sumForLoop' by measuring their Hertz values
// 'sumForLoop' is nearly 10 times faster than 'sumReduce'
console.log(Math.round(hz(sumReduce)));
console.log(Math.round(hz(sumForLoop)));
Output:
3584 2688
Flowchart:
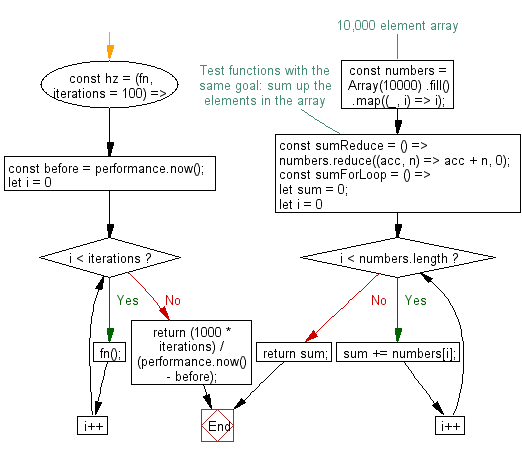
Live Demo:
See the Pen javascript-fundamental-exercise-218 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to check if the given number falls within the given range.
Next: Write a JavaScript program to calculate the Hamming distance between two values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-218.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics