JavaScript: Curry (curries) a function
JavaScript fundamental (ES6 Syntax): Exercise-46 with Solution
Curry a Function
Write a JavaScript program to curry (curries) a function
According to wiki.haskell.org "Currying is the process of transforming a function that takes multiple arguments into a function that takes just a single argument and returns another function if any arguments are still needed. f x y = g (x,y), however the curried form is usually more convenient because it allows partial application.
Note: Use recursion. If the number of provided arguments (args) is sufficient, call the passed function fn. Otherwise, return a curried function fn that expects the rest of the arguments. If you want to curry a function that accepts a variable number of arguments (a variadic function, e.g. Math.min()), you can optionally pass the number of arguments to the second parameter arity.
- Use recursion.
- If the number of provided arguments (args) is sufficient, call the passed function fn.
- Otherwise, use Function.prototype.bind() to return a curried function fn that expects the rest of the arguments.
- If you want to curry a function that accepts a variable number of arguments (a variadic function, e.g. Math.min()), you can optionally pass the number of arguments to the second parameter arity.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'curry' that takes a function 'fn', an arity 'arity', and optional arguments 'args'.
const curry = (fn, arity = fn.length, ...args) =>
// Check if the number of provided arguments is sufficient to invoke the function.
arity <= args.length ? fn(...args) : curry.bind(null, fn, arity, ...args);
// Test the 'curry' function with Math.pow and Math.min functions, and log the results.
console.log(curry(Math.pow)(2)(8)); // Output: 256
console.log(curry(Math.min, 3)(10)(50)(2)); // Output: 2
Output:
256 2
Flowchart:
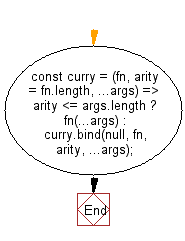
Live Demo:
See the Pen javascript-basic-exercise-1-46 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to split values into two groups, if an element in filter is truthy, the corresponding element in the collection belongs to the first group; otherwise, it belongs to the second group.
Next: Write a JavaScript program to perform a deep comparison between two values to determine if they are equivalent.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-46.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics