JavaScript: Split values into two groups with condition
JavaScript fundamental (ES6 Syntax): Exercise-45 with Solution
Write a JavaScript program to split values into two groups. If an element in the filter is true, the corresponding element in the collection belongs to the first group; otherwise, it belongs to the second group.
- Use Array.prototype.reduce() and Array.prototype.push() to add elements to groups, based on filter.
- If filter has a truthy value for any element, add it to the first group, otherwise add it to the second group.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'bifurcate' that takes an array 'arr' and a filter array 'filter' as input.
const bifurcate = (arr, filter) =>
// Reduce the array 'arr' using the filter array 'filter'.
arr.reduce((acc, val, i) =>
// Use the filter value at index 'i' to determine the destination array for the current value.
(acc[filter[i] ? 0 : 1].push(val), acc), [[], []]);
// Test the 'bifurcate' function with an array and a filter array, and log the result.
console.log(bifurcate(['beep', 'boop', 'foo', 'bar'], [true, true, false, true])); // Output: [['beep', 'boop', 'bar'], ['foo']]
Output:
[["beep","boop","bar"],["foo"]]
Flowchart:
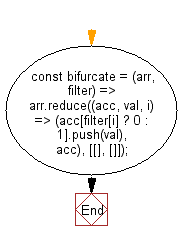
Live Demo:
See the Pen javascript-basic-exercise-1-45 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to remove falsey values from an given array.
Next: Write a JavaScript program to curry (curries) a function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics