JavaScript: Perform a deep comparison between two values to determine if they are equivalent
JavaScript fundamental (ES6 Syntax): Exercise-47 with Solution
Deep Comparison for Equivalence
Write a JavaScript program to perform a deep comparison between two values to determine if they are equivalent.
Note: Check if the two values are identical, if they are both Date objects with the same time, using Date.getTime() or if they are both non-object values with an equivalent value (strict comparison). Check if only one value is null or undefined or if their prototypes differ. If none of the above conditions are met, use Object.keys() to check if both values have the same number of keys, then use Array.every() to check if every key in the first value exists in the second one and if they are equivalent by calling this method recursively.
- Check if the two values are identical, if they are both Date objects with the same time, using Date.prototype.getTime() or if they are both non-object values with an equivalent value (strict comparison).
- Check if only one value is null or undefined or if their prototypes differ.
- If none of the above conditions are met, use Object.keys() to check if both values have the same number of keys.
- Use Array.prototype.every() to check if every key in a exists in b and if they are equivalent by calling equals() recursively.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'equals' that compares two values 'a' and 'b' for deep equality.
const equals = (a, b) => {
// Check if 'a' and 'b' are strictly equal.
if (a === b) return true;
// Check if 'a' and 'b' are instances of Date objects and have equal time values.
if (a instanceof Date && b instanceof Date) return a.getTime() === b.getTime();
// Check if either 'a' or 'b' is null, undefined, or not an object, and if they are equal.
if (!a || !b || (typeof a !== 'object' && typeof b !== 'object')) return a === b;
// Check if either 'a' or 'b' is null, undefined, or if their prototypes are not equal.
if (a === null || a === undefined || b === null || b === undefined || a.prototype !== b.prototype) return false;
// Get the keys of 'a'.
let keys = Object.keys(a);
// Check if the number of keys in 'a' is equal to the number of keys in 'b'.
if (keys.length !== Object.keys(b).length) return false;
// Check if every key-value pair in 'a' is equal to the corresponding key-value pair in 'b'.
return keys.every(k => equals(a[k], b[k]));
};
// Test the 'equals' function with two objects and log the result.
console.log(equals({ a: [2, { e: 3 }], b: [4], c: 'foo' }, { a: [2, { e: 3 }], b: [4], c: 'foo' })); // Output: true
Output:
true
Flowchart:
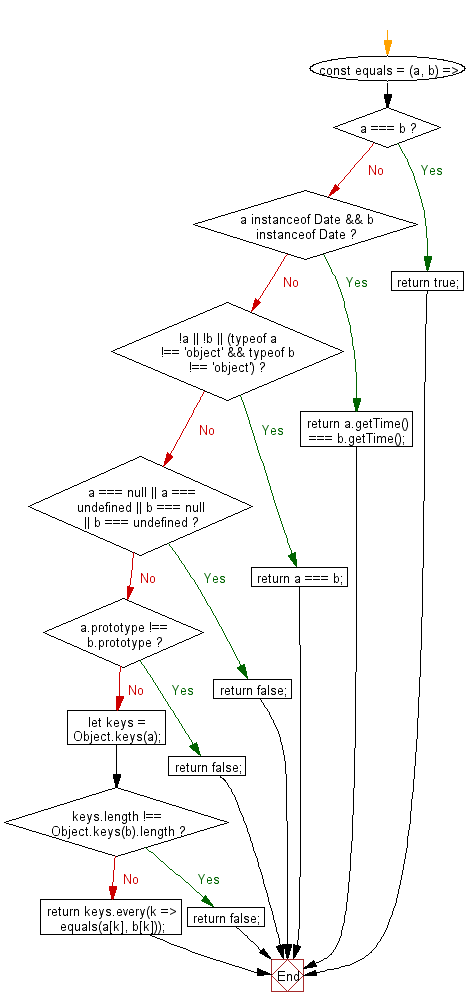
Live Demo:
See the Pen javascript-basic-exercise-1-47 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to curry (curries) a function
Next: Write a JavaScript program to get an array of function property names from own (and optionally inherited) enumerable properties of an object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-47.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics