JavaScript: Remove all falsy values from an object or array
JavaScript Array: Exercise-47 with Solution
Remove Falsey from Object or Array
Write a JavaScript program to remove all false values from an object or array.
- Use recursion.
- Initialize the iterable data, using Array.isArray(), Array.prototype.filter() and Boolean for arrays in order to avoid sparse arrays.
- Use Object.keys() and Array.prototype.reduce() to iterate over each key with an appropriate initial value.
- Use Boolean to determine the truthiness of each key's value and add it to the accumulator if it's truthy.
- Use typeof to determine if a given value is an object and call the function again to deeply compact it.
Sample Solution:
JavaScript Code :
// Source: https://bit.ly/3hEZdCl
// Function to compact an object by removing falsy values (null, false, 0, '', undefined)
const compactObject = val => {
// Use ternary operator to filter out falsy values for arrays, otherwise use the provided value
const data = Array.isArray(val) ? val.filter(Boolean) : val;
// Reduce the object to a compacted version, removing falsy values recursively
return Object.keys(data).reduce(
(acc, key) => {
const value = data[key];
// Check if the value is truthy before including it in the result
if (Boolean(value))
// Recursively compact object values, if applicable
acc[key] = typeof value === 'object' ? compactObject(value) : value;
return acc;
},
// Initialize the result as an empty array for arrays, otherwise an empty object
Array.isArray(val) ? [] : {}
);
};
// Sample object with various values including falsy ones
const obj = {
a: null,
b: false,
c: true,
d: 0,
e: 1,
f: '',
g: 'a',
h: [null, false, '', true, 1, 'a'],
i: { j: 0, k: false, l: 'a' }
};
// Output the result of compacting the object
console.log(compactObject(obj));
Output:
{"c":true,"e":1,"g":"a","h":[true,1,"a"],"i":{"l":"a"}}
Flowchart :
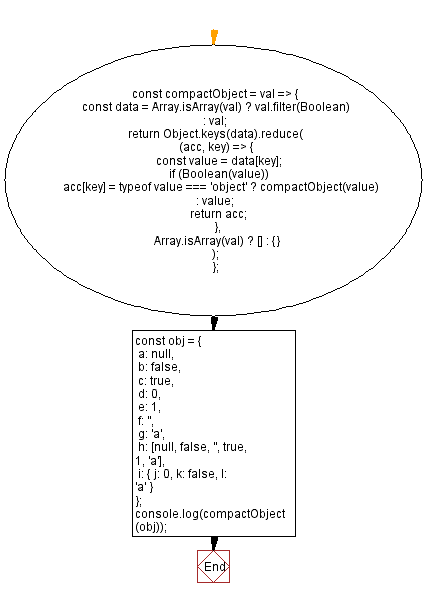
Live Demo :
See the Pen javascript-array-exercise-47 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that recursively removes all falsey values from an object and its nested arrays.
- Write a JavaScript function that filters an array to remove false, null, 0, "", undefined, and NaN values.
- Write a JavaScript function that traverses an object and deletes keys with falsey values, returning a cleaned object.
- Write a JavaScript function that generically removes falsey values from both arrays and objects, supporting nested structures.
Improve this sample solution and post your code through Disqus.
Previous: Permutations of an array elements.
.
Next: Check every numbers are prime or not in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.