JavaScript: Permutations of an array elements
JavaScript Array: Exercise-46 with Solution
Write a JavaScript program to generate all permutations of an array's elements (including duplicates).
- Use recursion.
- For each element in the given array, create all the partial permutations for the rest of its elements.
- Use Array.prototype.map() to combine the element with each partial permutation, then Array.prototype.reduce() to combine all permutations in one array.
- Base cases are for Array.prototype.length equal to 2 or 1.
- WARNING: This function's execution time increases exponentially with each array element. Anything more than 8 to 10 entries may cause your browser to hang as it tries to solve all the different combinations.
Sample Solution:
JavaScript Code :
// Source: https://bit.ly/3hEZdCl
// Function to generate permutations of an array
const permutations = arr => {
// Base case: if the array has 0 or 1 element, return the array
if (arr.length <= 2) return arr.length === 2 ? [arr, [arr[1], arr[0]]] : arr;
// Recursive case: generate permutations using reduce and recursion
return arr.reduce(
(acc, item, i) =>
acc.concat(
permutations([...arr.slice(0, i), ...arr.slice(i + 1)]).map(val => [
item,
...val,
])
),
[]
);
};
// Output the result of generating permutations for different arrays
console.log(permutations([1, 33, 5]));
console.log(permutations([1, 3, 5, 7]));
console.log(permutations([2, 4]));
Output:
[[1,33,5],[1,5,33],[33,1,5],[33,5,1],[5,1,33],[5,33,1]] [[1,3,5,7],[1,3,7,5],[1,5,3,7],[1,5,7,3],[1,7,3,5],[1,7,5,3],[3,1,5,7],[3,1,7,5],[3,5,1,7],[3,5,7,1],[3,7,1,5],[3,7,5,1],[5,1,3,7],[5,1,7,3],[5,3,1,7],[5,3,7,1],[5,7,1,3],[5,7,3,1],[7,1,3,5],[7,1,5,3],[7,3,1,5],[7,3,5,1],[7,5,1,3],[7,5,3,1]] [[2,4],[4,2]]
Flowchart :
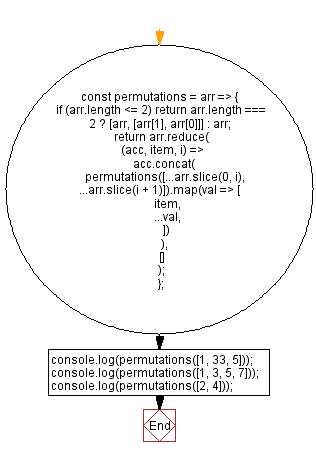
Live Demo :
See the Pen javascript-array-exercise-46 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Find all unique values in an array.
.
Next: Remove all falsy values from an object or array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics