JavaScript: Find all unique values in an array
JavaScript Array: Exercise-45 with Solution
Write a JavaScript program to find all the unique values in a set of numbers.
- Create a new Set() from the given array to discard duplicated values.
- Use the spread operator (...) to convert it back to an array
Sample Solution:
JavaScript Code :
// Function to return an array with unique elements using the Set data structure
const unique_Elements = arr => [...new Set(arr)];
// Output the result of applying unique_Elements to an array with duplicate elements
console.log(unique_Elements([1, 2, 2, 3, 4, 4, 5]));
// Output the result of applying unique_Elements to an array without duplicate elements
console.log(unique_Elements([1, 2, 3, 4, 5]));
// Output the result of applying unique_Elements to an array with negative and duplicate elements
console.log(unique_Elements([1, -2, -2, 3, 4, -5, -6, -5]));
Output:
[1,2,3,4,5] [1,2,3,4,5] [1,-2,3,4,-5,-6]
Flowchart :
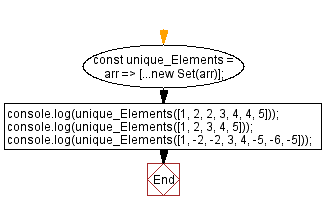
Live Demo :
See the Pen javascript-array-exercise-45 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Creates an object from an array, using the specified key and excluding it from each value.
.
Next: Permutations of an array elements
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-array-exercise-45.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics