JavaScript: Creates an object from an array, using the specified key and excluding it from each value
JavaScript Array: Exercise-44 with Solution
Object from Array with Key
Write a JavaScript function to create an object from an array, using the specified key and excluding it from each value.
- Use Array.prototype.reduce() to create an object from arr.
- Use object destructuring to get the value of the given key and the associated data and add the key-value pair to the object.
Sample Solution:
JavaScript Code :
// Source: https://bit.ly/3hEZdCl
// Function to create an object indexed by a specified key
const indexOn = (arr, key) =>
// Reduce the array to an object, using the specified key as the index
arr.reduce((obj, v) => {
// Destructure the input object, extracting the specified key and the remaining data
const { [key]: id, ...data } = v;
// Add the key-value pair to the object, using the specified key as the index
obj[id] = data;
// Return the updated object
return obj;
}, {});
// Output the result of indexing the array of objects by the 'id' property
console.log(indexOn([
{ id: 10, name: 'apple' },
{ id: 20, name: 'orange' }
], x => x.id));
Output:
{"undefined":{"id":20,"name":"orange"}}
Flowchart :
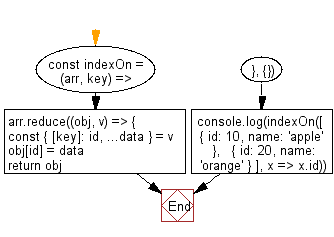
Live Demo :
See the Pen javascript-common-editor by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Ungrouping the elements in an array produced by zip.
Next: Find all unique values in an array
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-array-exercise-44.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics