JavaScript: Check every numbers are prime or not in an array
JavaScript Array: Exercise-48 with Solution
Write a JavaScript program that takes an array of integers and returns false if every number is not prime. Otherwise, return true.
Test Data:
([2,3,5,7]) -> true
([2,3,5,7,8]) -> false
Sample Solution-1:
JavaScript Code :
// Function to check if all numbers in an array are prime
function test(arr_nums) {
// Iterate through each number in the array
for (n of arr_nums) {
// Check if the number is equal to 1 or greater than 2 and divisible by 2
if (n == 1 || (n > 2 && n % 2 == 0))
// Return false if the condition is met for any number
return false;
}
// Return true if none of the numbers meet the specified condition
return true;
}
// Test the function with an array of prime numbers
nums = [2, 3, 5, 7];
console.log("Original array of integers: " + nums);
console.log("In the said array, check if every number is prime or not: " + test(nums));
// Test the function with an array containing a non-prime number (8)
nums = [2, 3, 5, 7, 8];
console.log("Original array of integers: " + nums);
console.log("In the said array, check if every number is prime or not: " + test(nums));
Output:
Original array of integers: 2,3,5,7 In the said array check every numbers are prime or not! true Original array of integers: 2,3,5,7,8 In the said array check every numbers are prime or not! false
Flowchart :
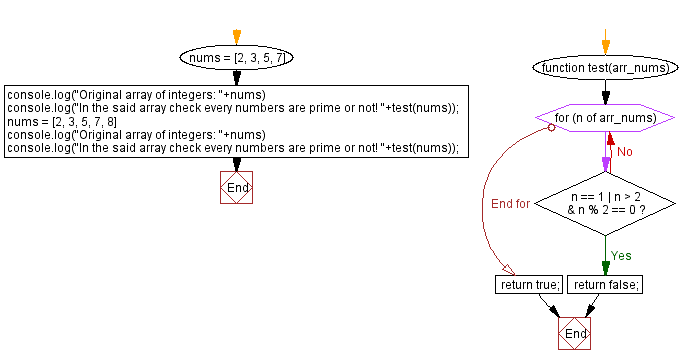
Live Demo :
See the Pen javascript-array-exercise-48 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code :
// Function to check if all numbers in an array are prime
function test(arr_nums) {
// Use the every method to check the primality of each number in the array
return arr_nums.every(n => !'1'.repeat(n).match(/^1?$|^(11+?)\1+$/));
}
// Test the function with an array of prime numbers
nums = [2, 3, 5, 7];
console.log("Original array of integers: " + nums);
console.log("In the said array, check if every number is prime or not: " + test(nums));
// Test the function with an array containing a non-prime number (8)
nums = [2, 3, 5, 7, 8];
console.log("Original array of integers: " + nums);
console.log("In the said array, check if every number is prime or not: " + test(nums));
Output:
Original array of integers: 2,3,5,7 In the said array check every numbers are prime or not! true Original array of integers: 2,3,5,7,8 In the said array check every numbers are prime or not! false
Flowchart :
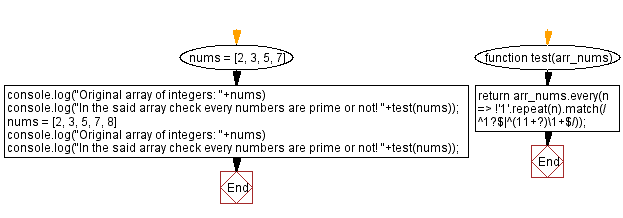
Live Demo :
See the Pen javascript-array-exercise-48-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Remove all falsy values from an object or array.
Next: Third smallest number of an array of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics