JavaScript: Get timezone offset in seconds
JavaScript Datetime: Exercise-40 with Solution
Timezone Offset (Seconds)
Write a JavaScript function to get the timezone offset in seconds.
Note : The offset for timezones west of UTC is always negative, and for those east of UTC is always positive.
Test Data :
dt = new Date();
console.log(timezone_offset_in_seconds(dt));
19800
Sample Solution:
JavaScript Code:
// Define a function timezone_offset_in_seconds that calculates the timezone offset in seconds for a given Date object dt
function timezone_offset_in_seconds(dt)
{
// Return the negated value of the timezone offset of the provided date multiplied by 60 (to convert minutes to seconds)
return -dt.getTimezoneOffset() * 60;
}
// Create a new Date object representing the current date and time
dt = new Date();
// Output the timezone offset in seconds for the current date and time
console.log(timezone_offset_in_seconds(dt));
// Create a new Date object representing November 1, 1989
dt = new Date(1989, 10, 1);
// Output the timezone offset in seconds for November 1, 1989
console.log(timezone_offset_in_seconds(dt));
Sample Output:
19800 19800
Explanation:
In the exercise above,
- The code defines a function called "timezone_offset_in_seconds()" that calculates the timezone offset in seconds for a given date.
- Inside the timezone_offset_in_seconds function:
- It calculates the timezone offset of the provided date using "dt.getTimezoneOffset()". This value represents the difference, in minutes, between the local timezone and UTC/GMT.
- It negates this value and multiplies it by 60 to convert minutes to seconds. The negation ensures that the returned value represents the offset from UTC/GMT rather than the local timezone.
- After defining the function, the code creates a new Date object "dt" representing the current date and time using 'new Date()'. It then calls the "timezone_offset_in_seconds()" function with this Date object and outputs the result (the timezone offset in seconds for the current date and time) to the console.
- Next, the code creates another new Date object "dt" representing November 1, 1989, using 'new Date(1989, 10, 1)'. It again calls the "timezone_offset_in_seconds()" function with this Date object and outputs the result to the console.
Flowchart:
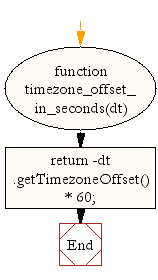
Live Demo:
See the Pen JavaScript - Get timezone offset in seconds-date-ex-40 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts the timezone offset from minutes (returned by getTimezoneOffset()) into seconds.
- Write a JavaScript function that validates a Date object and returns its timezone offset in seconds, handling negatives appropriately.
- Write a JavaScript function that compares the timezone offsets in minutes and seconds for a given Date.
- Write a JavaScript function that formats the timezone offset as a signed number of seconds.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get difference to Greenwich time (GMT) in hours.
Next: Write a JavaScript function to add specified years to a date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.