JavaScript: Get difference to Greenwich time in hours
JavaScript Datetime: Exercise-39 with Solution
GMT Difference
Write a JavaScript function to get the difference between Greenwich time (GMT) and in hours.
Test Data :
dt = new Date();
console.log(diff_to_GMT(dt));
"+05.500"
Sample Solution:
JavaScript Code:
// Define a function diff_to_GMT that takes a Date object dt as input
function diff_to_GMT(dt)
{
// Calculate the difference between the local timezone offset and GMT
// If the offset is negative, prepend '-' else prepend '+'
// Convert the offset to hours, ensuring a leading zero if the absolute value is less than 10
// Append '00' to represent minutes
return (-dt.getTimezoneOffset() < 0 ? '-' : '+') + (Math.abs(dt.getTimezoneOffset() / 60) < 10 ? '0' : '') + (Math.abs(dt.getTimezoneOffset() / 60)) + '00';
}
// Create a new Date object representing the current date and time
dt = new Date();
// Output the timezone difference to GMT for the current date and time
console.log(diff_to_GMT(dt));
// Create a new Date object representing November 1, 1989
dt = new Date(1989, 10, 1);
// Output the timezone difference to GMT for November 1, 1989
console.log(diff_to_GMT(dt));
Output:
+05.500 +05.500
Explanation:
In the exercise above,
- The code defines a function called "diff_to_GMT()" that calculates the timezone difference from GMT (Greenwich Mean Time) for a given date.
- Inside the diff_to_GMT function:
- It calculates the timezone offset of the provided date using "dt.getTimezoneOffset()". This value represents the difference, in minutes, between the local timezone and GMT.
- It checks if the timezone offset is negative:
- If it's negative, it means the local timezone is behind GMT, so it prepends a '-' sign to indicate this.
- If it's positive or zero, it means the local timezone is ahead of GMT, so it prepends a '+' sign.
- It then calculates the absolute value of the timezone offset divided by 60 to convert it to hours. If the absolute value is less than 10, it adds a leading '0' to ensure a two-digit representation.
- Finally, it appends '00' to represent the minutes component of the timezone offset.
- After defining the function, the code creates a new Date object "dt" representing the current date and time using 'new Date()'. It then calls the "diff_to_GMT()" function with this Date object and outputs the result (the timezone difference to GMT for the current date and time) to the console.
- Next, the code creates another new Date object "dt" representing November 1, 1989, using 'new Date(1989, 10, 1)'. It again calls the "diff_to_GMT()" function with this Date object and outputs the result to the console.
Flowchart:
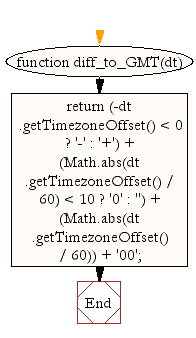
Live Demo:
See the Pen JavaScript - Get difference to Greenwich time in hours-date-ex-39 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the difference between local time and GMT in hours, formatted with a plus or minus sign.
- Write a JavaScript function that extracts the timezone offset from a Date object and converts it to a string like "+05.500".
- Write a JavaScript function that computes the GMT difference and returns it with two decimal precision.
- Write a JavaScript function that validates the Date input and handles non-standard offsets gracefully.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to find whether or not the date is in daylights savings time.
Next: Write a JavaScript function to get timezone offset in seconds.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.