JavaScript: Add specified years to a date
JavaScript Datetime: Exercise-41 with Solution
Add Years to Date
Write a JavaScript function to add specified years to a date.
Test Data:
dt = new Date(2014,10,2);
console.log(add_years(dt, 10).toString());
Output:
"Sat Nov 02 2024 00:00:00 GMT+0530 (India Standard Time)"
Sample Solution:
JavaScript Code:
// Define a function add_years that adds a specified number of years (n) to a given Date object (dt)
function add_years(dt,n)
{
// Set the full year of the provided date to the result of adding n years to its current full year
return new Date(dt.setFullYear(dt.getFullYear() + n));
}
// Create a new Date object representing the current date and time
dt = new Date();
// Output the result of adding 10 years to the current date and time
console.log(add_years(dt, 10).toString());
// Create a new Date object representing November 2, 2014
dt = new Date(2014,10,2);
// Output the result of adding 10 years to November 2, 2014
console.log(add_years(dt, 10).toString());
Output:
Tue Jun 20 2028 16:40:21 GMT+0530 (India Standard Time) Sat Nov 02 2024 00:00:00 GMT+0530 (India Standard Time)
Explanation:
In the exercise above,
- The code defines a function called "add_years()" that takes two parameters:
- dt: A Date object representing a specific date.
- n: The number of years to add to the provided date.
- Inside the add_years function:
- It uses the "setFullYear()" method of the Date object ("dt") to modify its year component. It adds the specified number of years ('n') to the current year of the Date object.
- The "setFullYear()" method updates the year part of the date and returns the number of milliseconds since January 1, 1970, 00:00:00 UTC, representing the updated date.
- After defining the function, the code creates a new Date object "dt" representing the current date and time using 'new Date()'.
- It then calls the "add_years()" function with this Date object and the number 10 as arguments to add 10 years to the current date and time.
- Finally, it outputs the result of adding 10 years to the current date and time using console.log().
- Next, the code creates another new Date object "dt" representing November 2, 2014, using new Date(2014,10,2).
- It again calls the "add_years()" function with this Date object and the number 10 as arguments to add 10 years to November 2, 2014.
- Finally, it outputs the result of adding 10 years to November 2, 2014, using console.log().
Flowchart:
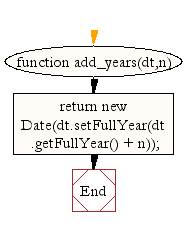
Live Demo:
See the Pen JavaScript - Add specified years to a date-date-ex-41 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that adds a specified number of years to a Date object using setFullYear() and returns the new date.
- Write a JavaScript function that handles leap years correctly when adding years to a date.
- Write a JavaScript function that validates the input years before adding them to a Date object.
- Write a JavaScript function that formats the updated Date object into a readable string after adding years.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get timezone offset in seconds.
Next: Write a JavaScript function to add specified weeks to a date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.