JavaScript Product Class: Calculate total price with warranty
JavaScript OOP: Exercise-10 with Solution
Product and PersonalCareProduct Classes with Warranty
Write a JavaScript program that creates a class called Product with properties for product ID, name, and price. Include a method to calculate the total price by multiplying the price by the quantity. Create a subclass called PersonalCareProduct that inherits from the Product class and adds an additional property for the warranty period. Override the total price calculation method to include the warranty period. Create an instance of the PersonalCareProduct class and calculate its total price.
Note: This program shows the concept of inheritance and method overriding in JavaScript object-oriented programming.
Sample Solution:
JavaScript Code:
class Product {
constructor(id, name, price) {
this.id = id;
this.name = name;
this.price = price;
}
calculateTotalPrice(quantity) {
return this.price * quantity;
}
}
class PersonalCareProduct extends Product {
constructor(id, name, price, warrantyPeriod) {
super(id, name, price);
this.warrantyPeriod = warrantyPeriod;
}
calculateTotalPrice(quantity) {
const totalPrice = super.calculateTotalPrice(quantity);
return totalPrice + this.warrantyPeriod;
}
}
// Create an instance of the PersonalCareProduct class
const personalCareProduct = new PersonalCareProduct(1, 'Shampoo', 10, 2);
// Calculate the total price
const totalPrice = personalCareProduct.calculateTotalPrice(3);
console.log(`Total Price: $${totalPrice}`);
Sample Output:
"Total Price: $32"
Note: Executed on JS Bin
Explanation:
In the above exercise -
First, we create the "Product" class with properties for product ID, name, and price. It includes a method called calculateTotalPrice() that takes a quantity parameter and calculates the total price by multiplying the price by the quantity.
A subclass called "PersonalCareProduct" is created using the extends keyword to inherit from the "Product" class. It adds an additional property called warrantyPeriod. The PersonalCareProduct class overrides the calculateTotalPrice() method to include the warranty period in the total price calculation.
An instance of the "PersonalCareProduct" class is created with a product ID of 1, name of "Shampoo", price of $10, and warranty period of 2. The calculateTotalPrice() method is called on the personalCareProduct instance with a quantity of 3, resulting in a total price of $32.
Flowchart:
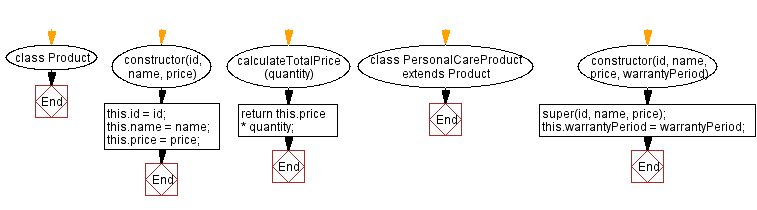
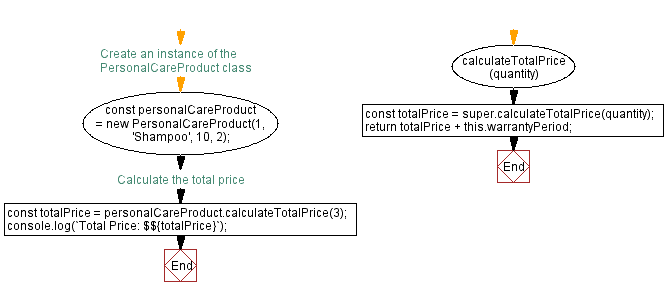
Live Demo:
See the Pen javascript-oop-exercise-10 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript class called Product with properties for product ID, name, and price, including a method to calculate the total price based on quantity; extend it with a PersonalCareProduct class that adds a warranty period and overrides the total price calculation to include warranty costs.
- Write a JavaScript class called Product that includes a method to apply discounts, then extend it with PersonalCareProduct to offer bundled warranty services.
- Write a JavaScript class called PersonalCareProduct that extends Product and validates that the warranty period is within acceptable limits.
- Write a JavaScript class called PersonalCareProduct that extends Product, adds a method to compare warranty periods with another product, and returns the one with the longer warranty.
Improve this sample solution and post your code through Disqus
OOP Exercises Previous: Manage bank branches.
OOP Exercises Next: Account management operations.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.