JavaScript Bank Class: Manage bank branches
JavaScript OOP: Exercise-9 with Solution
Bank Class with Branch Management
Write a JavaScript program that creates a class called Bank with properties for bank names and branches. Include methods to add a branch, remove a branch, and display all branches. Create an instance of the Bank class and perform operations to add and remove branches.
Sample Solution:
JavaScript Code:
class Bank {
constructor() {
this.bankName = '';
this.branches = [];
}
addBranch(branch) {
this.branches.push(branch);
console.log(`${branch} added successfully.`);
}
removeBranch(branch) {
const index = this.branches.indexOf(branch);
if (index !== -1) {
this.branches.splice(index, 1);
console.log(`Branch ${branch} removed successfully.`);
}
else {
console.log(`Branch "${branch}" does not exist.`);
}
}
displayBranches() {
if (this.branches.length === 0) {
console.log('No branches available.');
} else {
console.log('List of Bank Branches:');
this.branches.forEach((branch, index) => {
console.log(`${index + 1}. ${branch}`);
});
}
}
}
// Create an instance of the Bank class
const bank = new Bank();
// Add branches
bank.addBranch('Branch A');
bank.addBranch('Branch B');
bank.addBranch('Branch C');
// Display all branches
bank.displayBranches();
// Remove a branch
bank.removeBranch('Branch B');
// Display all branches again
bank.displayBranches();
Sample Output:
"Branch A added successfully." "Branch B added successfully." "Branch C added successfully." "List of Bank Branches:" "1. Branch A" "2. Branch B" "3. Branch C" "Branch Branch B removed successfully." "List of Bank Branches:" "1. Branch A" "2. Branch C"
Note: Executed on JS Bin
Explanation:
In the above exercise -
First, we create a "Bank" class with properties for bank names and branches. It includes methods to add a branch, remove a branch, and display all branches.
An instance of the "Bank" class is created with an empty bankName and branches array. The "addBranch()" method adds a new branch to the branches array and logs a success message. The "removeBranch()" method removes a specified branch from the branches array if it exists and logs a success message. Otherwise, it displays a message indicating that the branch does not exist. The "displayBranches()" method displays a list of branches if there are any, otherwise, it displays a message indicating that there are no branches available.
Finally the program creates an instance of the "Bank" class and performs operations to add three branches, display all branches, remove one branch, and display the updated list of branches.
Flowchart:
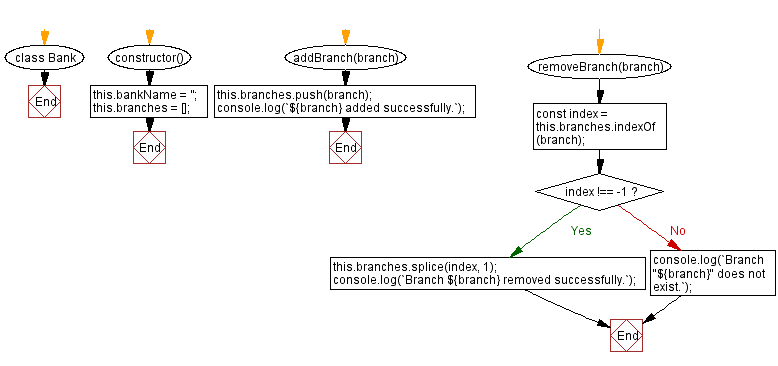
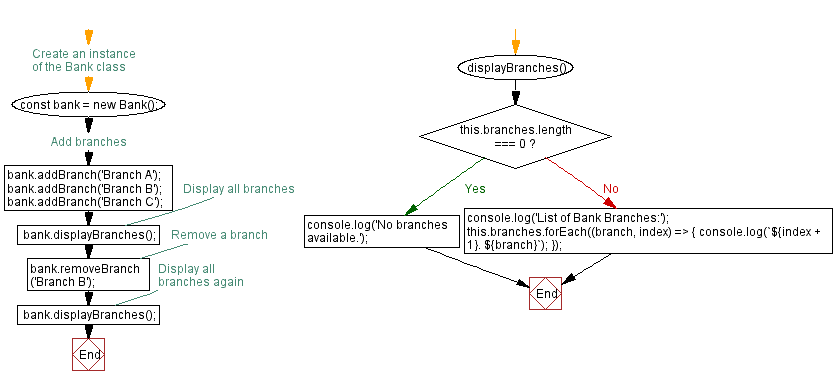
Live Demo:
See the Pen javascript-oop-exercise-9 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript class called Bank with properties for bank name and branches (an array), including methods to add, remove, and display branches.
- Write a JavaScript class called Bank that maintains a list of branches and includes a method to search for a branch by name.
- Write a JavaScript class called Bank that implements methods to add multiple branches at once and to sort the branch names alphabetically.
- Write a JavaScript class called Bank that tracks the history of branch additions and removals, then provides a method to display the change log.
Improve this sample solution and post your code through Disqus
OOP Exercises Previous: Make sounds with color.
OOP Exercises Next: Calculate total price with warranty.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.