JavaScript Animal and Dog Classes: Make sounds with color
JavaScript OOP: Exercise-8 with Solution
Write a JavaScript program that creates a class called 'Animal' with properties for species and sound. Include a method to make the animal's sound. Create a subclass called 'Dog' that inherits from the 'Animal' class and adds an additional property for color. Override the make sound method to include the dog's color. Create an instance of the 'Dog' class and make it make its sound.
Sample Solution:
JavaScript Code:
class Animal {
constructor(species, sound) {
this.species = species;
this.sound = sound;
}
makeSound() {
console.log(this.sound);
}
}
class Dog extends Animal {
constructor(species, sound, color) {
super(species, sound);
this.color = color;
}
makeSound() {
super.makeSound();
console.log(`Color: ${this.color}`);
}
}
// Create an instance of the Dog class
const dog = new Dog('Dog', 'Woof woof!', 'Brown');
// Make the dog make its sound
dog.makeSound();
Sample Output:
"Woof woof!" "Color: Brown"
Note: Executed on JS Bin
Explanation:
In the above exercise -
We create a class called "Animal" with properties for species and sound. It includes a "makeSound()" method that logs the animal's sound.
The "Dog" class is a subclass of "Animal" and adds a property for color. It overrides the "makeSound()" method to first call the parent class's "makeSound()" method using super.makeSound(), and then logs the dog's color.
An instance of the "Dog" class is created with the species "Dog", the sound "Woof woof!", and the color "Brown". The "makeSound()" method is called for the dog, and it logs the dog's sound and color.
Flowchart:
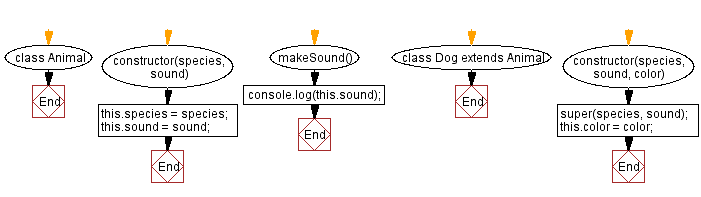
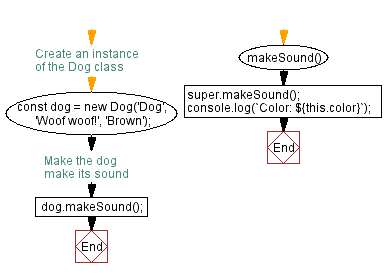
Live Demo:
See the Pen javascript-oop-exercise-8 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
OOP Exercises Previous: Display book details with price.
OOP Exercises Next: Manage bank branches.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics