JavaScript Book and Ebook Classes: Display book details with price
JavaScript OOP: Exercise-7 with Solution
Book and Ebook Classes with Price
Write a JavaScript program that creates a class `Book` with properties for title, author, and publication year. Include a method to display book details. Create a subclass called 'Ebook' that inherits from the 'Book' class and includes an additional property for book price. Override the display method to include the book price. Create an instance of the 'Ebook' class and display its details.
Sample Solution:
JavaScript Code:
class Book {
constructor(title, author, publicationYear) {
this.title = title;
this.author = author;
this.publicationYear = publicationYear;
}
displayDetails() {
console.log(`Title: ${this.title}`);
console.log(`Author: ${this.author}`);
console.log(`Publication Year: ${this.publicationYear}`);
}
}
class Ebook extends Book {
constructor(title, author, publicationYear, price) {
super(title, author, publicationYear);
this.price = price;
}
displayDetails() {
super.displayDetails();
console.log(`Price: $${this.price}`);
}
}
// Create an instance of the Ebook class
const ebook = new Ebook('Don Quixote', 'Miguel de Cervantes', 1605, 21.49);
// Display the ebook details
ebook.displayDetails();
Sample Output:
"Title: Don Quixote" "Author: Miguel de Cervantes" "Publication Year: 1605" "Price: $21.49"
Note: Executed on JS Bin
Explanation:
In the above exercise -
We create a class called "Book" with properties for title, author, and publication year. It includes a "displayDetails()" method that displays the book's title, author, and publication year.
The "Ebook" class is a subclass of "Book" and adds a property for book price. It overrides the "displayDetails()" method to first call the parent class's displayDetails() method using super.displayDetails(), and then displays the book price specific to ebooks.
An instance of the Ebook class is created with the title "Don Quixote", the author "Miguel de Cervantes", a publication year of 1605, and a price of $21.49. The "displayDetails()" method is called for the ebook, and it displays the ebook's details including the overridden information specific to ebooks.
Flowchart:
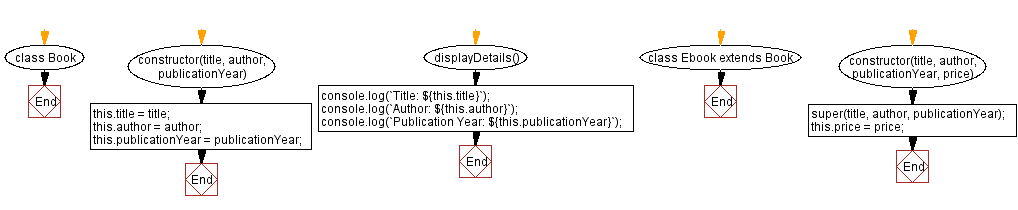
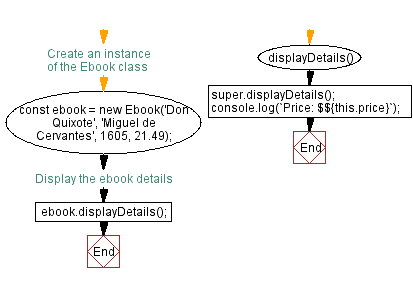
Live Demo:
See the Pen javascript-oop-exercise-7 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript class called Book with properties for title, author, and publication year, and a method to display details; extend it with an Ebook class that adds price and file size properties.
- Write a JavaScript class called Book that includes a method to update the publication year, and extend it with an Ebook class that overrides the display method to show digital details.
- Write a JavaScript class called Book that implements a method to compare two books by publication year; extend it with an Ebook class to perform price comparisons.
- Write a JavaScript class called Ebook that extends Book and includes a method to apply a discount to the price, ensuring the discount does not exceed a specified percentage.
Improve this sample solution and post your code through Disqus
OOP Exercises Previous: Calculate annual salary.
OOP Exercises Next: Make sounds with color.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.