JavaScript Employee and Manager Classes: Calculate annual salary
JavaScript OOP: Exercise-6 with Solution
Employee and Manager Classes with Salary Calculation
Write a JavaScript program that creates a class called 'Employee' with properties for name and salary. Include a method to calculate annual salary. Create a subclass called 'Manager' that inherits from the 'Employee' class and adds an additional property for department. Override the annual salary calculation method to include bonuses for managers. Create two instances of the 'Manager' class and calculate their annual salary.
Sample Solution:
JavaScript Code:
class Employee {
constructor(name, salary) {
this.name = name;
this.salary = salary;
console.log(`Name of the Employee: ${name}`);
console.log(`Monthly Salary: $${salary}`);
}
calculateAnnualSalary() {
return this.salary * 12;
}
}
class Manager extends Employee {
constructor(name, salary, department) {
super(name, salary);
this.department = department;
}
calculateAnnualSalary() {
const baseSalary = super.calculateAnnualSalary();
const bonus = 0.1;
console.log(`Bonus (10% of the base salary): ${bonus}`);
// Bonus calculation for managers
return (bonus * baseSalary) + baseSalary;
}
}
// Create an instance of the Manager class
const manager1 = new Manager('Angela Luca', 5000, 'Marketing');
const annualSalary1 = manager1.calculateAnnualSalary();
console.log(`Manager: ${manager1.name}`);
console.log(`Department: ${manager1.department}`);
console.log(`Annual Salary: $${annualSalary1}`);
// Create another instance of the Manager class
const manager2 = new Manager('Jonelle Rozaliya', 5500, 'Marketing');
const annualSalary2 = manager2.calculateAnnualSalary();
console.log(`Manager: ${manager2.name}`);
console.log(`Department: ${manager2.department}`);
console.log(`Annual Salary: $${annualSalary2}`);
Sample Output:
"Name of the Employee: Angela Luca" "Monthly Salary: $5000" "Bonus (10% of the base salary): 0.1" "Manager: Angela Luca" "Department: Marketing" "Annual Salary: $66000" "Name of the Employee: Jonelle Rozaliya" "Monthly Salary: $5500" "Bonus (10% of the base salary): 0.1" "Manager: Jonelle Rozaliya" "Department: Marketing" "Annual Salary: $72600"
Note: Executed on JS Bin
Explanation:
In the above code we first create an "Employee" class with properties for name and salary. It includes a "calculateAnnualSalary()" method that calculates the annual salary by multiplying the monthly salary by 12.
The "Manager" class is a subclass of "Employee" and adds an additional property for each department. It overrides the "calculateAnnualSalary()" method to include a bonus of 10% of the base salary for managers.
Two instances of the "Manager" class are created with a given name and salary and a department of "Marketing". The "calculateAnnualSalary()" method is called for the manager, and the calculated annual salary and details of the employees are displayed on the console.
Flowchart:
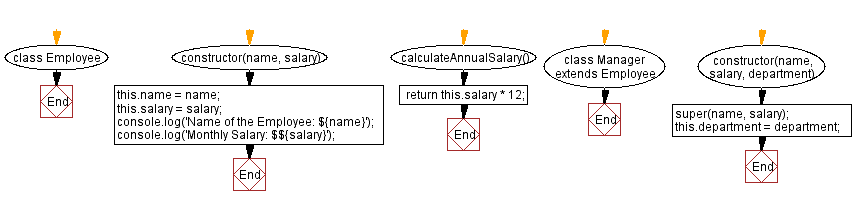
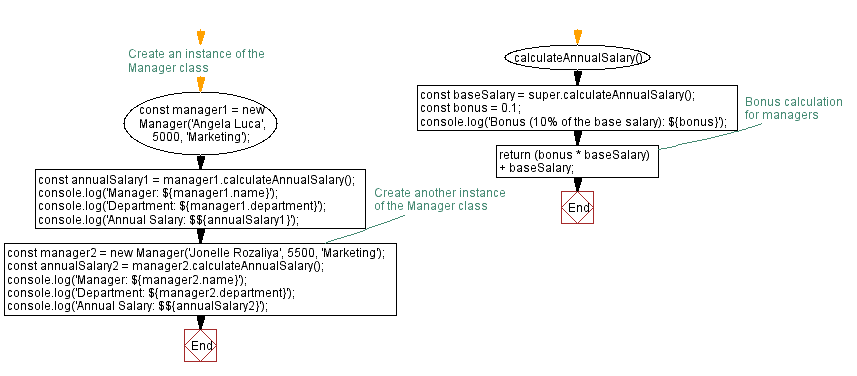
Live Demo:
See the Pen javascript-oop-exercise-6 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript class called Employee with properties for name and salary, including a method to compute the annual salary; then create a Manager subclass that adds a bonus property and overrides the salary calculation.
- Write a JavaScript class called Employee that includes a method to increase the salary by a given percentage; extend it with a Manager class that incorporates department allowances.
- Write a JavaScript class called Employee that displays employee details, and create a Manager subclass with a static method to compare manager salaries.
- Write a JavaScript class called Manager that extends Employee, adds a department property, and overrides the salary calculation to include performance bonuses.
Improve this sample solution and post your code through Disqus
OOP Exercises Previous: Calculate area for circle and triangle.
OOP Exercises Next: Display book details with price.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.