JavaScript Shape Class: Calculate area for circle and triangle
JavaScript OOP: Exercise-5 with Solution
Shape, Circle, and Triangle Classes with Area Calculation
Write a JavaScript program that creates a class called 'Shape' with a method to calculate the area. Create two subclasses, 'Circle' and 'Triangle', that inherit from the 'Shape' class and override the area calculation method. Create an instance of the 'Circle' class and calculate its area. Similarly, do the same for the 'Triangle' class.
Sample Solution:
JavaScript Code:
class Shape {
calculateArea() {
throw new Error("Method 'calculateArea()' must be overridden in subclasses");
}
}
class Circle extends Shape {
constructor(radius) {
super();
this.radius = radius;
}
calculateArea() {
return Math.PI * this.radius * this.radius;
}
}
class Rectangle extends Shape {
constructor(width, height) {
super();
this.width = width;
this.height = height;
}
calculateArea() {
return this.width * this.height;
}
}
// Create an instance of the Circle class
const circle = new Circle(7);
const circleArea = circle.calculateArea();
console.log(`Circle Area: ${circleArea}`);
// Create an instance of the Rectangle class
const rectangle = new Rectangle(8, 9);
const rectangleArea = rectangle.calculateArea();
console.log(`Rectangle Area: ${rectangleArea}`);
Sample Output:
"Circle Area: 153.93804002589985" "Rectangle Area: 72"
Note: Executed on JS Bin
Explanation:
In the above exercise -
In the code above, the "Shape" class has a "calculateArea()" method that throws an error, indicating that it must be overridden by subclasses. This enforces that Shape subclasses must provide their own implementation of the "calculateArea()" method.
The "Circle" class extends "Shape" and overrides the "calculateArea()" method to calculate the area of a circle based on its radius using the formula Math.PI * radius^2.
The "Rectangle" class also extends "Shape" and provides its own implementation of the calculateArea() method to calculate the area of a rectangle based on its width and height using the formula width * height.
Finally, instances of 'Circle' and 'Rectangle' are created. Their respective "calculateArea()" methods are called to calculate and display the circle and rectangle areas.
Flowchart:
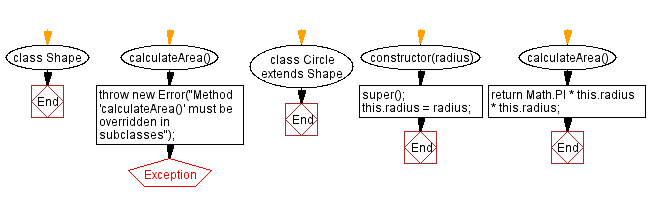
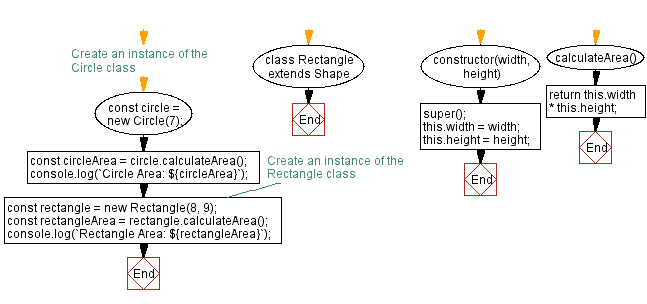
Live Demo:
See the Pen javascript-oop-exercise-5 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript abstract class called Shape with an area method; then create subclasses Circle and Triangle that override the area method using the proper formulas.
- Write a JavaScript class called Circle that extends Shape, validates the radius input, and calculates the area using Math.PI.
- Write a JavaScript class called Triangle that extends Shape, accepts base and height, and calculates its area; then add a method to compute the perimeter given side lengths.
- Write a JavaScript program that creates an array of mixed Shape objects (circles and triangles) and uses polymorphism to compute and display each shape’s area.
Improve this sample solution and post your code through Disqus
OOP Exercises Previous: Manage account balance.
OOP Exercises Next: Calculate annual salary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.