JavaScript Bank Account Class: Manage account balance
JavaScript OOP: Exercise-4 with Solution
Write a JavaScript program that creates a class called "BankAccount" with properties for account number and balance. Include methods to deposit and withdraw money from the account. Create some instances of the "BankAccount" class, deposit some money, and withdraw a portion of it.
Sample Solution:
JavaScript Code:
class BankAccount {
constructor(accountNumber, balance) {
this.accountNumber = accountNumber;
this.balance = balance;
console.log(`A/c No.: ${accountNumber}`);
console.log(`Opening Balance: $${balance}`);
}
deposit(amount) {
this.balance += amount;
console.log(`Deposited: $${amount}`);
}
withdraw(amount) {
if (amount <= this.balance) {
this.balance -= amount;
console.log(`Withdrawn: $${amount}`);
}
else
{
console.log(`Want to withdrawn: $${amount}`);
console.log('Insufficient balance');
}
}
displayBalance() {
console.log(`Account Balance: $${this.balance}`);
}
}
// Create an instance of the BankAccount class
const account = new BankAccount('SB-123', 1500);
// Deposit money into the account
account.deposit(500);
// Withdraw money from the account
account.withdraw(400);
// Display the account balance
account.displayBalance();
// Withdraw money from the account
account.withdraw(1800);
// Display the account balance
account.displayBalance();
Sample Output:
"A/c No.: SB-123" "Opening Balance: $1500" "Deposited: $500" "Withdrawn: $400" "Account Balance: $1600" "Want to withdrawn: $1800" "Insufficient balance" "Account Balance: $1600"
Note: Executed on JS Bin
Explanation:
In the above exercise,
- We first create a "BankAccount" class with properties for account number and balance. It includes methods "deposit()" and "withdraw()" to deposit and withdraw money from the account respectively.
- An instance of the BankAccount class is created using the new keyword and assigned to the variable account. The constructor is called with arguments 'SB-123' for the account number and 1500 for the initial balance.
- The deposit method of the account object is called with an argument of $500 to deposit $500 into the account.
- The withdraw method of the account object is called with an argument of $400 to withdraw $400 from the account. Since the balance is sufficient, the withdrawal is processed.
- The displayBalance method of the account object displays the current account balance.
- The withdraw method of the account object is called again, this time with an argument of 1800, which is more than the current balance. The withdrawal is not processed due to insufficient balance, and an appropriate message is logged.
- The displayBalance method is called again to display the updated account balance.
Flowchart:
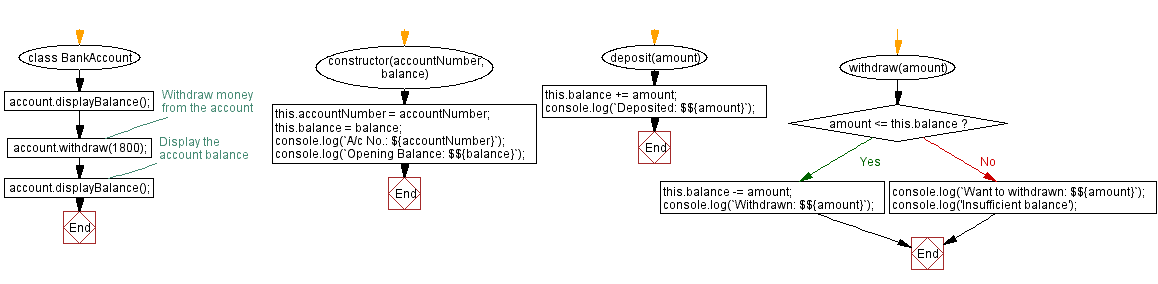
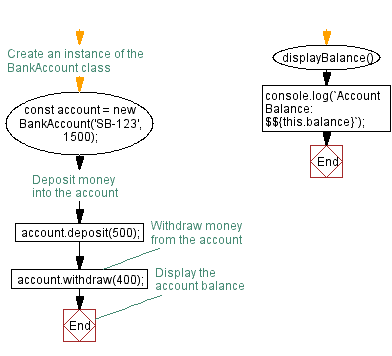
Live Demo:
See the Pen javascript-oop-exercise-4 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
OOP Exercises Previous: Vehicle and car subclass with details.
OOP Exercises Next: Calculate area for circle and triangle.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics