JavaScript Class: Vehicle and car subclass with details
JavaScript OOP: Exercise-3 with Solution
Vehicle and Car Classes with Inheritance
Write a JavaScript program that creates a class called 'Vehicle' with properties for make, model, and year. Include a method to display vehicle details. Create a subclass called 'Car' that inherits from the 'Vehicle' class and includes an additional property for the number of doors. Override the display method to include the number of doors.
Sample Solution:
JavaScript Code:
class Vehicle {
constructor(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
displayDetails() {
console.log(`Make: ${this.make}`);
console.log(`Model: ${this.model}`);
console.log(`Year: ${this.year}`);
}
}
class Car extends Vehicle {
constructor(make, model, year, doors) {
super(make, model, year);
this.doors = doors;
}
displayDetails() {
super.displayDetails();
console.log(`Doors: ${this.doors}`);
}
}
// Create an instance of the Vehicle class
const vehicle = new Vehicle('Ford', 'F-150', 2020);
// Display vehicle details
console.log('Vehicle Details:');
vehicle.displayDetails();
// Create an instance of the Car class
const car = new Car('Honda', 'Accord', 2023, 4);
// Display car details
console.log('\nCar Details:');
car.displayDetails();
Sample Output:
"Vehicle Details:" "Make: Ford" "Model: F-150" "Year: 2020" "Car Details:" "Make: Honda" "Model: Accord" "Year: 2023" "Doors: 4"
Note: Executed on JS Bin
Explanation:
In the above exercise,
We create a class called "Vehicle" with properties for make, model, and year. It includes a method "displayDetails()" to display vehicle details. A subclass called "Car" is created, which inherits from the “Vehicle” class and includes an additional property for the number of doors. The "displayDetails() " method is overridden in the "Car" class to include the number of doors. An instance of the "Vehicle " class and an instance of the "Car" class are created, and their details are displayed using the "displayDetails()" method.
Flowchart:
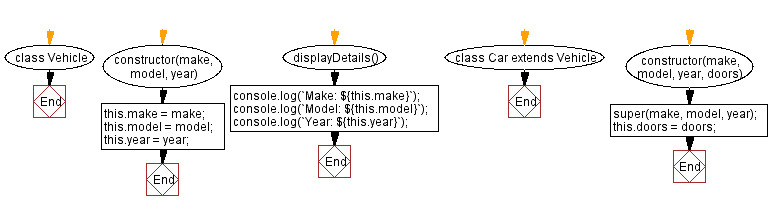
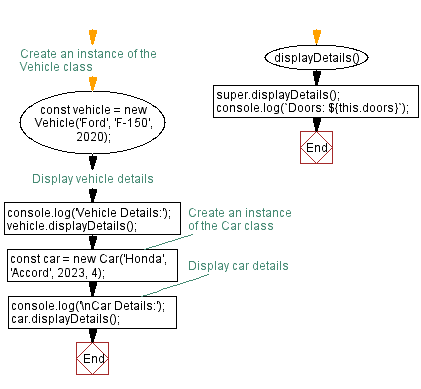
Live Demo:
See the Pen javascript-oop-exercise-3 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript class called Vehicle with properties for make, model, and year, and a method to return a formatted description; then create a Car subclass that adds a property for the number of doors and overrides the description method.
- Write a JavaScript class called Vehicle that includes a method to calculate the vehicle's age from the current year; extend it with a Car class that also stores fuel efficiency.
- Write a JavaScript class called Vehicle that implements a static method to compare the model year of two vehicles; extend it with a Car class that adds seating capacity.
- Write a JavaScript class called Vehicle that uses constructor inheritance with super(), then create a Car subclass that adds a method to determine if the car is vintage (over 25 years old).
Go to:
PREV : Rectangle Class with Area and Perimeter.
NEXT : BankAccount Class with Deposit and Withdrawal.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.