JavaScript Class: Rectangle with area and perimeter methods
JavaScript OOP: Exercise-2 with Solution
Rectangle Class with Area and Perimeter
Write a JavaScript program to create a class called 'Rectangle' with properties for width and height. Include two methods to calculate rectangle area and perimeter. Create an instance of the 'Rectangle' class and calculate its area and perimeter.
Sample Solution:
JavaScript Code:
class Rectangle {
constructor(width, height) {
this.width = width;
this.height = height;
}
calculateArea() {
return this.width * this.height;
}
calculatePerimeter() {
return 2 * (this.width + this.height);
}
}
// Create an instance of the Rectangle class
const rectangle = new Rectangle(12, 10);
// Calculate area and perimeter of the rectangle
const area = rectangle.calculateArea();
const perimeter = rectangle.calculatePerimeter();
// Display the results
console.log(`Rectangle Area: ${area}`);
console.log(`Rectangle Perimeter: ${perimeter}`);
Sample Output:
"Rectangle Area: 120" "Rectangle Perimeter: 44"
Note: Executed on JS Bin
Explanation:
The above exercise creates a "Rectangle" class with properties for width and height. It includes two methods, "calculateArea()" and "calculatePerimeter()", to calculate the area and perimeter respectively. An instance of the "Rectangle" class is created with width 12 and height 10, and then the area and perimeter are calculated using the respective methods. The results are then displayed on the console.
Flowchart:
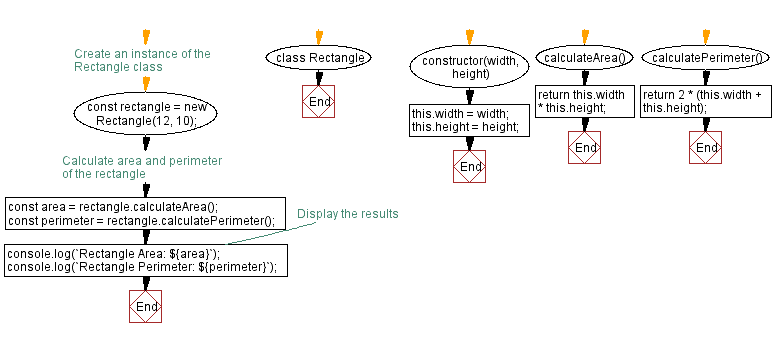
Live Demo:
See the Pen javascript-oop-exercise-2 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript class called Rectangle that calculates area and perimeter, and throws an error if width or height is non-numeric.
- Write a JavaScript class called Rectangle that supports updating its dimensions after instantiation, automatically recalculating area and perimeter.
- Write a JavaScript class called Rectangle that includes a static method to compare two rectangles based on their area.
- Write a JavaScript class called Rectangle that draws a textual representation of the rectangle in the console using repeated characters.
Improve this sample solution and post your code through Disqus
OOP Exercises Previous: Person with name, age, and country properties.
OOP Exercises Next: Vehicle and car subclass with details.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.