JavaScript Bank Account Class: Account management operations
JavaScript OOP: Exercise-11 with Solution
Write a JavaScript program that creates a class called BankAccount with properties for account number, account holder name, and balance. Include methods to deposit, withdraw, and transfer money between accounts. Create multiple instances of the BankAccount class and perform operations such as depositing, withdrawing, and transferring money.
Sample Solution:
JavaScript Code:
class BankAccount {
constructor(accountNumber, accountHolderName, balance) {
this.accountNumber = accountNumber;
this.accountHolderName = accountHolderName;
this.balance = balance;
}
deposit(amount) {
this.balance += amount;
console.log(`Amount $${amount} deposited into account ${this.accountNumber}.`);
}
withdraw(amount) {
if (amount <= this.balance) {
this.balance -= amount;
console.log(`Amount $${amount} withdrawn from account ${this.accountNumber}.`);
} else {
console.log(`Insufficient balance in account ${this.accountNumber}.`);
}
}
transfer(amount, recipientAccount) {
if (amount <= this.balance) {
this.balance -= amount;
recipientAccount.deposit(amount);
console.log(`Amount $${amount} transferred from account ${this.accountNumber} to account ${recipientAccount.accountNumber}.`);
} else {
console.log(`Insufficient balance in account ${this.accountNumber} for transfer.`);
}
}
displayBalance() {
console.log(`Account ${this.accountNumber} balance: $${this.balance}`);
}
}
// Create multiple instances of the BankAccount class
const account1 = new BankAccount('SB-012', 'Karishma Hedy', 2000);
const account2 = new BankAccount('SB-019', 'Phokas Intan', 3000);
// Transactions on the bank accounts
account1.displayBalance(); // Account SB-012 balance: $2000
account2.displayBalance(); // Account SB-019 balance: $3000
account1.deposit(500); // Amount $500 deposited into account SB-012.
account1.displayBalance(); // Account SB-012 balance: $2500
account1.withdraw(200); // Amount $200 withdrawn from account SB-012.
account1.displayBalance(); // Account SB-012 balance: $2300
account1.transfer(700, account2); // Amount $700 transferred from account SB-01 to account SB-019.
account1.displayBalance(); // Account SB-012 balance: $1600
account2.displayBalance(); // Account SB-019 balance: $3700
Sample Output:
"Account SB-012 balance: $2000" "Account SB-019 balance: $3000" "Amount $500 deposited into account SB-012." "Account SB-012 balance: $2500" "Amount $200 withdrawn from account SB-012." "Account SB-012 balance: $2300" "Amount $700 deposited into account SB-019." "Amount $700 transferred from account SB-012 to account SB-019." "Account SB-012 balance: $1600" "Account SB-019 balance: $3700"
Note: Executed on JS Bin
Explanation:
First we create a "BankAccount" class with properties for account number, account holder name, and balance. It includes methods to deposit, withdraw, and transfer money between accounts.
Multiple instances of the "BankAccount" class are created (account1 and account2). Operations such as depositing, withdrawing, and transferring money are performed on these accounts. The "displayBalance()" method displays the balance of each account after operations.
Flowchart:
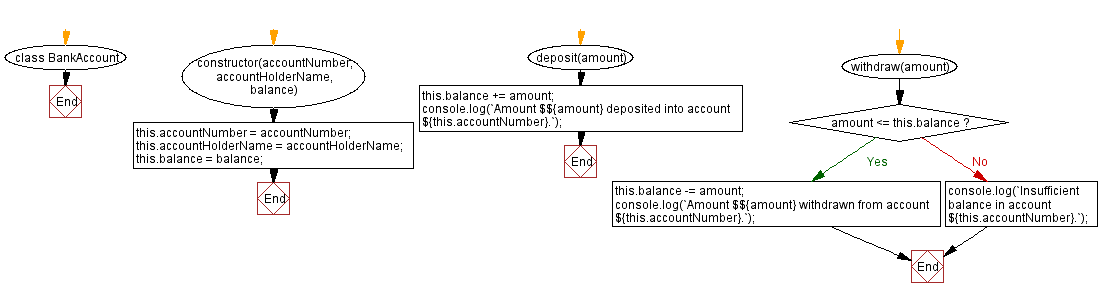
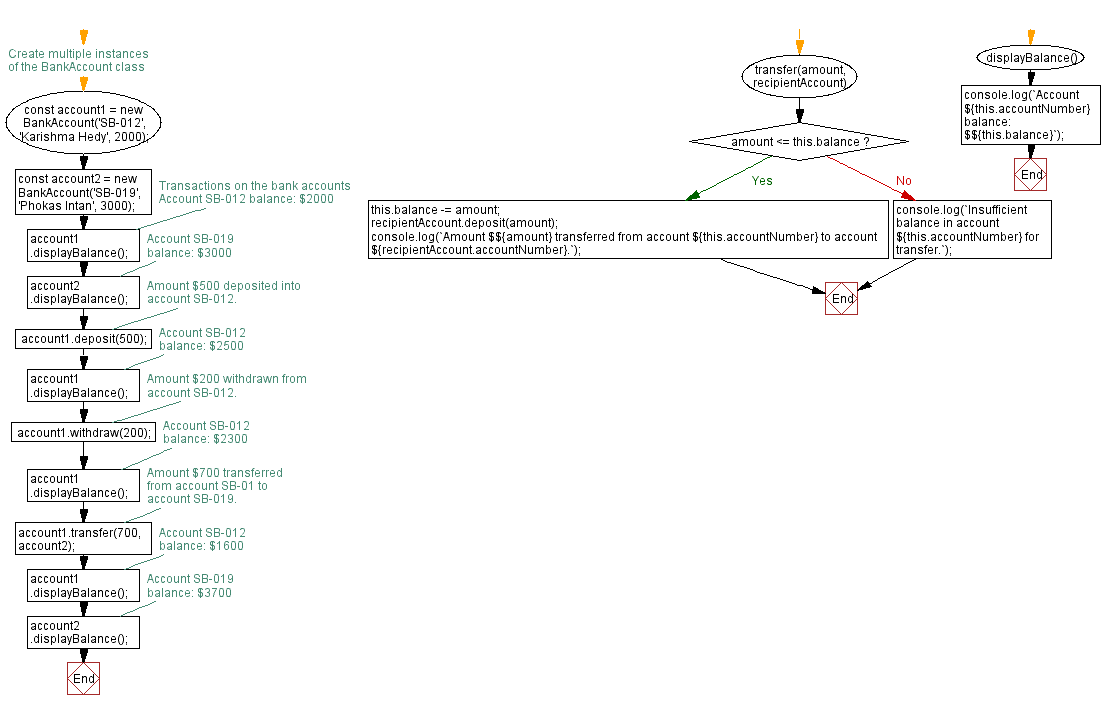
Live Demo:
See the Pen javascript-oop-exercise-11 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
OOP Exercises Previous: Calculate total price with warranty.
OOP Exercises Next: Department of management and operations.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics