JavaScript University Class: Department of management and operations
JavaScript OOP: Exercise-12 with Solution
University Class with Department Management
Write a JavaScript program that creates a class called University with properties for university name and departments. Include methods to add a department, remove a department, and display all departments. Create an instance of the University class and add and remove departments.
Sample Solution:
JavaScript Code:
class University {
constructor(name) {
this.name = name;
this.departments = [];
}
addDepartment(department) {
this.departments.push(department);
console.log(`Department "${department}" added to ${this.name}.`);
}
removeDepartment(department) {
const index = this.departments.indexOf(department);
if (index !== -1) {
this.departments.splice(index, 1);
console.log(`Department "${department}" removed from ${this.name}.`);
} else {
console.log(`Department "${department}" does not exist in ${this.name}.`);
}
}
displayDepartments() {
console.log(`Departments in ${this.name}:`);
if (this.departments.length === 0) {
console.log('No departments available.');
} else {
this.departments.forEach((department, index) => {
console.log(`${index + 1}. ${department}`);
});
}
}
}
// Create an instance of the University class
const university = new University('ABC University');
// Perform operations on the university
university.displayDepartments(); // Departments in ABC University: No departments available.
university.addDepartment('Science');
university.addDepartment('Mathematics');
university.addDepartment('Physics');
university.displayDepartments();
// Departments in ABC University:
// 1. Computer Science
// 2. Mathematics
// 3. Physics
university.removeDepartment('Mathematics');
university.displayDepartments();
// Departments in ABC University:
// 1. Science
// 2. Physics
university.removeDepartment('Chemistry');
// Department "Chemistry" does not exist in ABC University.
Sample Output:
"Departments in ABC University:" "No departments available." "Department \"Science\" added to ABC University." "Department \"Mathematics\" added to ABC University." "Department \"Physics\" added to ABC University." "Departments in ABC University:" "1. Science" "2. Mathematics" "3. Physics" "Department \"Mathematics\" removed from ABC University." "Departments in ABC University:" "1. Science" "2. Physics" "Department \"Chemistry\" does not exist in ABC University."
Note: Executed on JS Bin
Explanation:
First we create a "University" class with properties for university name and departments. It includes methods to add a department, remove a department, and display all departments.
An instance of the University class (university) is created, and operations such as adding and removing departments are performed. The "displayDepartments()" method is used to display all departments of the university after each operation.
Flowchart:
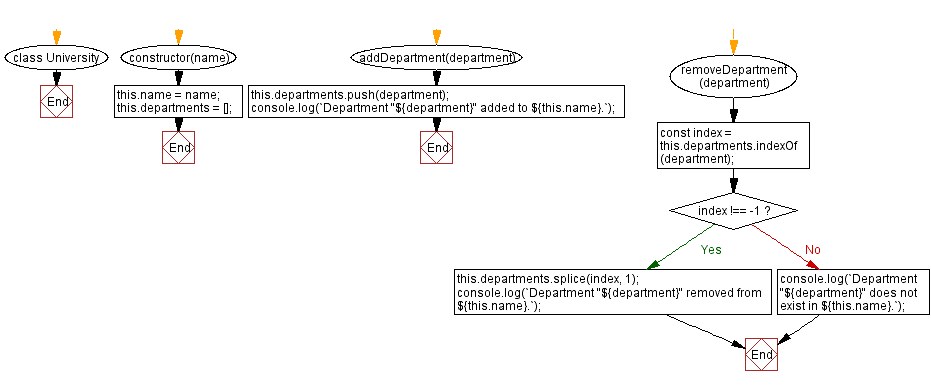
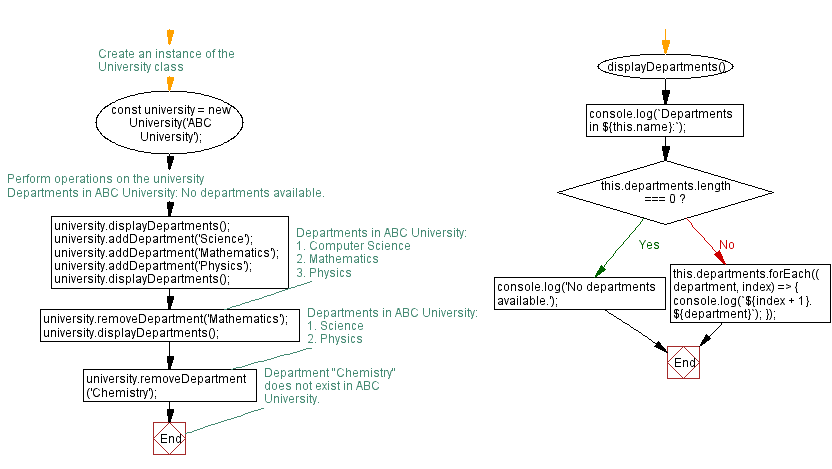
Live Demo:
See the Pen javascript-oop-exercise-12 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript class called University with properties for the university name and an array of departments, including methods to add, remove, and list departments.
- Write a JavaScript class called University that allows adding departments and then searching for a department by name using a case-insensitive match.
- Write a JavaScript class called University that maintains a sorted list of departments and includes a method to merge departments with similar names.
- Write a JavaScript class called University that tracks department additions and removals, and provides a method to export the department list as a formatted string.
Improve this sample solution and post your code through Disqus
OOP Exercises Previous: Account management operations.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.